用atmega128写一个电子时钟程序
时间: 2023-09-30 08:06:08 浏览: 124
好的,下面是一个简单的电子时钟程序,使用ATmega128单片机实现。
```c
#include <avr/io.h>
#include <avr/interrupt.h>
#include <util/delay.h>
#define F_CPU 16000000UL
#define BAUD 9600
#define MYUBRR F_CPU/16/BAUD-1
volatile uint16_t milliseconds = 0;
volatile uint8_t seconds = 0;
volatile uint8_t minutes = 0;
volatile uint8_t hours = 0;
void USART_Init( unsigned int ubrr ) {
UBRR0H = (unsigned char)(ubrr>>8);
UBRR0L = (unsigned char)ubrr;
UCSR0B = (1<<TXEN0)|(1<<RXEN0);
UCSR0C = (1<<UCSZ00)|(1<<UCSZ01);
}
void USART_Transmit( unsigned char data ) {
while ( !( UCSR0A & (1<<UDRE0)) );
UDR0 = data;
}
ISR(TIMER0_COMPA_vect) {
milliseconds++;
if (milliseconds >= 1000) {
seconds++;
milliseconds = 0;
if (seconds >= 60) {
minutes++;
seconds = 0;
if (minutes >= 60) {
hours++;
minutes = 0;
if (hours >= 24) {
hours = 0;
}
}
}
}
}
void printDigit(uint8_t digit) {
USART_Transmit(digit/10 + '0');
USART_Transmit(digit%10 + '0');
}
int main(void) {
USART_Init(MYUBRR);
TCCR0A = (1<<WGM01);
TCCR0B = (1<<CS02)|(1<<CS00);
OCR0A = 156;
TIMSK0 = (1<<OCIE0A);
sei();
while (1) {
printDigit(hours);
USART_Transmit(':');
printDigit(minutes);
USART_Transmit(':');
printDigit(seconds);
USART_Transmit('\n');
_delay_ms(1000);
}
return 0;
}
```
该程序使用AVR的定时器来实现电子时钟的计时功能,并通过串口输出来显示当前时间。其中,定时器0的计时周期为1ms,使用中断来实现时间的累加,同时使用串口输出函数将时间通过串口发送出去。
请注意,该程序仅供参考,可能需要根据具体硬件和应用场景进行修改和优化。
阅读全文
相关推荐




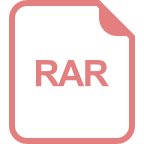










