@ExtendWith(SpringExtension.class) @SpringBootTest
时间: 2024-09-10 09:10:40 浏览: 106
`@ExtendWith(SpringExtension.class)` 是Spring Boot测试框架的一部分,它用于扩展`@SpringBootTest`的功能。通常情况下,当你在测试类上使用`@SpringBootTest`时,这个注解会自动加载一个完整的Spring应用上下文(ApplicationContext),这样你在测试中就可以注入依赖并模拟真实的业务环境。
然而,从提供的引用来看[^1]^2],`@SpringBootTest`已经默认包含了对`SpringExtension`的扩展,这意味着你不再需要显式地在测试类上添加`@ExtendWith(SpringExtension.class)`,因为它的功能已经被整合到`@SpringBootTest`之中了。这可以让你的测试配置更加简洁,直接使用`@SpringBootTest`即可开始测试,而不需要额外声明其他扩展。
例如,一个简单的测试类可能看起来像这样:
```java
@SpringBootTest
public class MyServiceTest {
// 测试方法...
}
```
这里的`@SpringBootTest`已经隐含了`SpringExtension`的行为,所以无需单独引入。测试类会自动启动一个与生产环境类似的测试上下文,供测试方法使用。
相关问题
@Configuration public class ElasticSearchConfig { public static final RequestOptions COMMON_OPTIONS; static { RequestOptions.Builder builder = RequestOptions.DEFAULT.toBuilder(); // builder.addHeader("Authorization", "Bearer " + TOKEN); // builder.setHttpAsyncResponseConsumerFactory( // new HttpAsyncResponseConsumerFactory // .HeapBufferedResponseConsumerFactory(30 * 1024 * 1024 * 1024)); COMMON_OPTIONS = builder.build(); } @Bean public RestHighLevelClient esRestClient(){ RestHighLevelClient client = new RestHighLevelClient( RestClient.builder(new HttpHost("127.0.0.1", 9200, "http"))); return client; } } @ExtendWith(SpringExtension.class) @ContextConfiguration(classes = ElasticSearchConfig.class) public class YourTest { // 测试方法... } 里面怎么写
你的代码已经基本正确,只需要做一些小的改动。
首先,你需要导入必要的包:
```java
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit.jupiter.SpringExtension;
```
然后,你需要修改`YourTest`类中的测试方法,以使用`RestHighLevelClient`进行测试。例如:
```java
@SpringBootTest(classes = ElasticSearchConfig.class)
@ExtendWith(SpringExtension.class)
public class YourTest {
@Autowired
private RestHighLevelClient restHighLevelClient;
@Test
public void testElasticsearchConnection() throws IOException {
// 编写你的测试逻辑...
}
}
```
在测试方法中,你可以使用`restHighLevelClient`来执行与Elasticsearch相关的操作。例如,你可以测试与Elasticsearch的连接是否正常,或者执行一些简单的索引和搜索操作。
记得在测试方法上加上`@Test`注解来标识它是一个测试方法。然后,你可以使用JUnit运行这个测试类来执行测试。
注意:在运行测试之前,确保你的Elasticsearch服务器正在运行,并且与配置类中指定的主机和端口一致。
springboot测试类怎么建
在Spring Boot中,测试类是用来对应用程序的各个组件进行单元测试的。
要建立一个Spring Boot测试类,需要遵循以下步骤:
1. 在测试源代码目录(src/test/java)下创建一个与被测试类相对应的包结构。
2. 在该包下创建一个新的Java类,并添加`@RunWith(SpringRunner.class)`注解,它告诉JUnit运行器使用Spring的测试上下文。
3. 如果你使用JUnit 5,则需要使用`@ExtendWith(SpringExtension.class)`注解替代`@RunWith(SpringRunner.class)`注解。
4. 在测试类上使用`@SpringBootTest`注解,它会加载整个Spring应用程序上下文以进行集成测试。
5. 根据需要添加其他注解,如`@AutoConfigureMockMvc`(用于集成测试Web层)、`@DataJpaTest`(用于集成测试JPA层)等。
6. 创建测试方法,并添加必要的断言和测试逻辑。
以下是一个示例:
```java
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testMyService() {
// 进行测试逻辑
// 使用断言进行验证
}
}
```
请注意,上述示例中的`MyService`是一个被测试的服务类,`@Autowired`注解用于将其注入到测试类中。
这只是一个简单的示例,你可以根据具体的需求和场景来编写你的测试类。希望对你有所帮助!
阅读全文
相关推荐
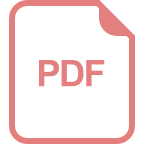
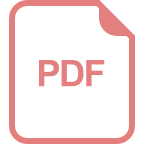
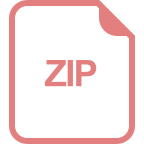
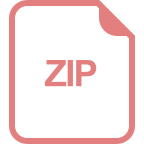
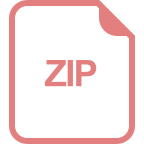
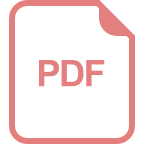
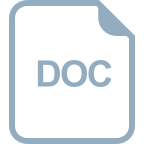
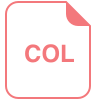
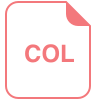
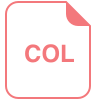
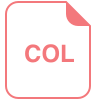
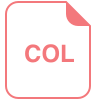
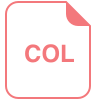
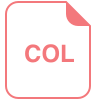
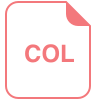
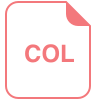
