Write a readable and writable program using Android's internal storage, and display it below after inputting text for reading and writing
时间: 2024-02-04 17:01:55 浏览: 103
Sure, here's a simple Android app that allows you to read and write text to the device's internal storage:
MainActivity.java:
```
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
public class MainActivity extends AppCompatActivity {
EditText editText;
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.edit_text);
textView = findViewById(R.id.text_view);
loadText();
}
public void saveText(View view) {
String text = editText.getText().toString();
FileOutputStream outputStream;
try {
outputStream = openFileOutput("text_file", Context.MODE_PRIVATE);
outputStream.write(text.getBytes());
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void loadText() {
FileInputStream inputStream;
try {
File file = new File(getFilesDir(), "text_file");
if (file.exists()) {
inputStream = openFileInput("text_file");
InputStreamReader isr = new InputStreamReader(inputStream);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
textView.setText(sb.toString());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
activity_main.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save"
android:onClick="saveText"/>
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
```
This app has an EditText for inputting text, a Button for saving the text, and a TextView for displaying the text that has been saved. When the "Save" button is clicked, the app writes the text to a file named "text_file" in the device's internal storage. When the app is started, it loads the text from the file if it exists and displays it in the TextView.
To run the app, you can create a new Android project in Android Studio and copy the code above into the appropriate files. Then you can run the app on an emulator or a physical Android device.
阅读全文
相关推荐
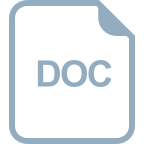
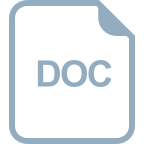
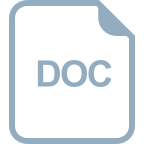
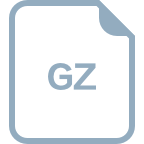
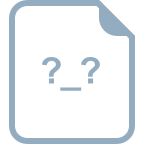
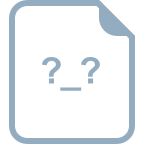
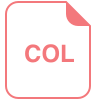










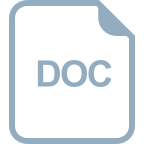
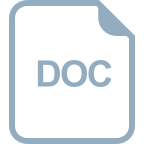