操作系统进程管理模拟
时间: 2023-12-03 08:04:15 浏览: 36
以下是一个简单的操作系统进程管理模拟的Python代码,其中包括进程的创建、阻塞、唤醒和撤销等基本操作:
```python
class PCB:
def __init__(self, pid, priority, state):
self.pid = pid
self.priority = priority
self.state = state
class ProcessQueue:
def __init__(self):
self.queue = []
def add_process(self, process):
self.queue.append(process)
def remove_process(self, pid):
for process in self.queue:
if process.pid == pid:
self.queue.remove(process)
return True
return False
def get_highest_priority_process(self):
if len(self.queue) == 0:
return None
highest_priority_process = self.queue[0]
for process in self.queue:
if process.priority > highest_priority_process.priority:
highest_priority_process = process
return highest_priority_process
ready_queue = ProcessQueue()
blocked_queue = ProcessQueue()
while True:
command = input("请输入命令:")
if command == "create":
pid = input("请输入进程ID:")
priority = int(input("请输入进程优先级:"))
state = "ready"
process = PCB(pid, priority, state)
ready_queue.add_process(process)
elif command == "block":
pid = input("请输入进程ID:")
if ready_queue.remove_process(pid):
process = PCB(pid, 0, "blocked")
blocked_queue.add_process(process)
else:
print("进程不存在或不在就绪队列中")
elif command == "wake":
pid = input("请输入进程ID:")
if blocked_queue.remove_process(pid):
process = PCB(pid, 0, "ready")
ready_queue.add_process(process)
else:
print("进程不存在或不在阻塞队列中")
elif command == "kill":
pid = input("请输入进程ID:")
if ready_queue.remove_process(pid):
print("进程已从就绪队列中撤销")
elif blocked_queue.remove_process(pid):
print("进程已从阻塞队列中撤销")
else:
print("进程不存在")
elif command == "schedule":
process = ready_queue.get_highest_priority_process()
if process is not None:
print("当前运行进程:", process.pid)
ready_queue.remove_process(process.pid)
else:
print("就绪队列为空")
else:
print("无效命令")
```
相关推荐
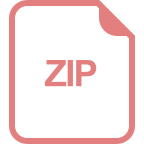














