python如何在字符串中检索关键词
时间: 2024-11-25 16:21:13 浏览: 16
在Python中,你可以使用内置的字符串方法或正则表达式模块(re模块)来检索字符串中的关键词。这里列举两种常见的方法:
**1. 使用in关键字和find()方法**
如果你想要查找的是简单关键词是否存在,可以直接使用`in`关键字和`str.find()`方法:
```python
string = "Hello, world!"
keyword = "world"
if keyword in string:
print(f"Keyword '{keyword}' found.")
else:
print(f"'{keyword}' not found in the string.")
```
`str.find(keyword)`会返回关键词第一次出现的位置,如果找不到则返回-1。
**2. 使用replace()或split()方法**
如果你想替换所有的关键词或者将关键词分割出来,可以考虑`str.replace()`或`str.split()`方法:
```python
string = "I love coding in Python"
keyword = "Python"
# 替换所有出现的关键词
new_string = string.replace(keyword, "Java")
print(new_string)
# 将关键词分割成列表
words = string.split(" ")
keywords_list = [word for word in words if word == keyword]
print(keywords_list)
```
**3. 使用正则表达式(re模块)**
对于更复杂的搜索模式,如匹配特定的字符组合或者使用正则表达式的规则,可以使用`re.search()`或`re.findall()`等函数:
```python
import re
pattern = r"\bPython\b" # 匹配完整的单词 "Python"
match = re.search(pattern, string)
if match:
print("Match found at position:", match.start())
else:
print("No match")
all_matches = re.findall(pattern, string)
print("All matches:", all_matches)
```
阅读全文
相关推荐
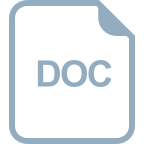
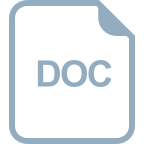
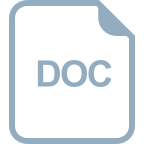
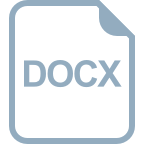
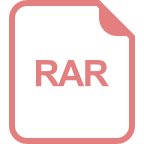
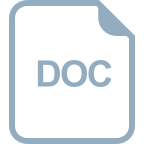
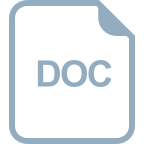
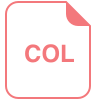
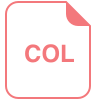









