python 字符串检索
时间: 2023-10-01 12:10:16 浏览: 134
在 Python 中,可以使用字符串方法 `find()` 或 `index()` 来检索字符串中的子串。这两种方法都可以返回子串第一次出现的索引,如果子串不存在,则返回 -1(`find()`)或抛出 `ValueError` 异常(`index()`)。
例如,假设要在字符串 `s = "hello world"` 中查找子串 `"world"`:
```python
s = "hello world"
idx = s.find("world")
if idx != -1:
print(f"Substring found at index {idx}")
else:
print("Substring not found")
```
输出结果为:
```
Substring found at index 6
```
`index()` 方法与 `find()` 方法类似,只是当子串不存在时会抛出异常。如果要避免异常,可以使用 `in` 关键字进行检查:
```python
s = "hello world"
if "world" in s:
idx = s.index("world")
print(f"Substring found at index {idx}")
else:
print("Substring not found")
```
输出结果与之前相同:
```
Substring found at index 6
```
相关问题
Python英文字符串检索每个字母的数量并输出
可以使用 Python 中的 Counter 函数来实现字符串中每个字母的数量检索,示例如下:
```python
from collections import Counter
# 定义字符串
s = 'Hello, World!'
# 使用 Counter 函数统计每个字母的数量
counter = Counter(s)
# 输出每个字母的数量
for letter, count in counter.items():
if letter.isalpha():
print(f"{letter}: {count}")
```
输出结果为:
```
H: 1
e: 1
l: 3
o: 2
W: 1
r: 1
d: 1
```
这里使用了 `isalpha()` 函数来判断字母,避免输出非字母字符的数量。
python检索字符串去重
在Python中,有几种方法可以用于从字符串列表中去除重复的内容:
1. **使用set()**:Set数据结构自动去重,你可以将字符串列表转换为集合,然后再转换回列表。
```python
string_list = ['a', 'b', 'c', 'a', 'd', 'c']
unique_strings = list(set(string_list))
```
2. **使用dict.fromkeys()**:利用字典的键值唯一特性,遍历列表并添加元素到字典,然后取字典的键。
```python
string_list = ['a', 'b', 'c', 'a', 'd', 'c']
unique_strings = list(dict.fromkeys(string_list))
```
3. **使用列表推导式**:通过比较每个元素是否已经在新列表中,只保留第一次出现的元素。
```python
string_list = ['a', 'b', 'c', 'a', 'd', 'c']
unique_strings = [x for i, x in enumerate(string_list) if x not in string_list[:i]]
```
4. **使用collections模块的Counter**:对于计数排序,这也很有用。
```python
from collections import Counter
string_list = ['a', 'b', 'c', 'a', 'd', 'c']
unique_strings = [item for item, count in Counter(string_list).items() if count == 1]
```
以上都是常见的去重方法,选择哪种取决于你的具体需求和数据规模。
阅读全文
相关推荐
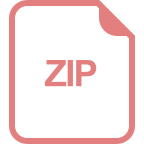
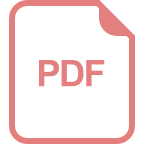
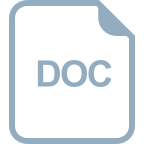
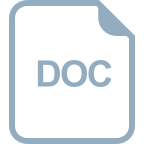
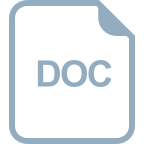
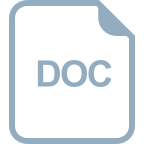
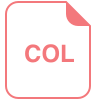
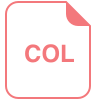
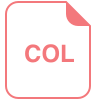
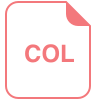
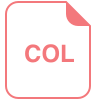
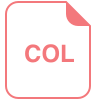
