揭秘Python字符串处理计数技巧:解锁字符串中字母个数的秘密
发布时间: 2024-06-25 08:25:16 阅读量: 74 订阅数: 29 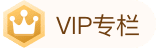
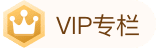
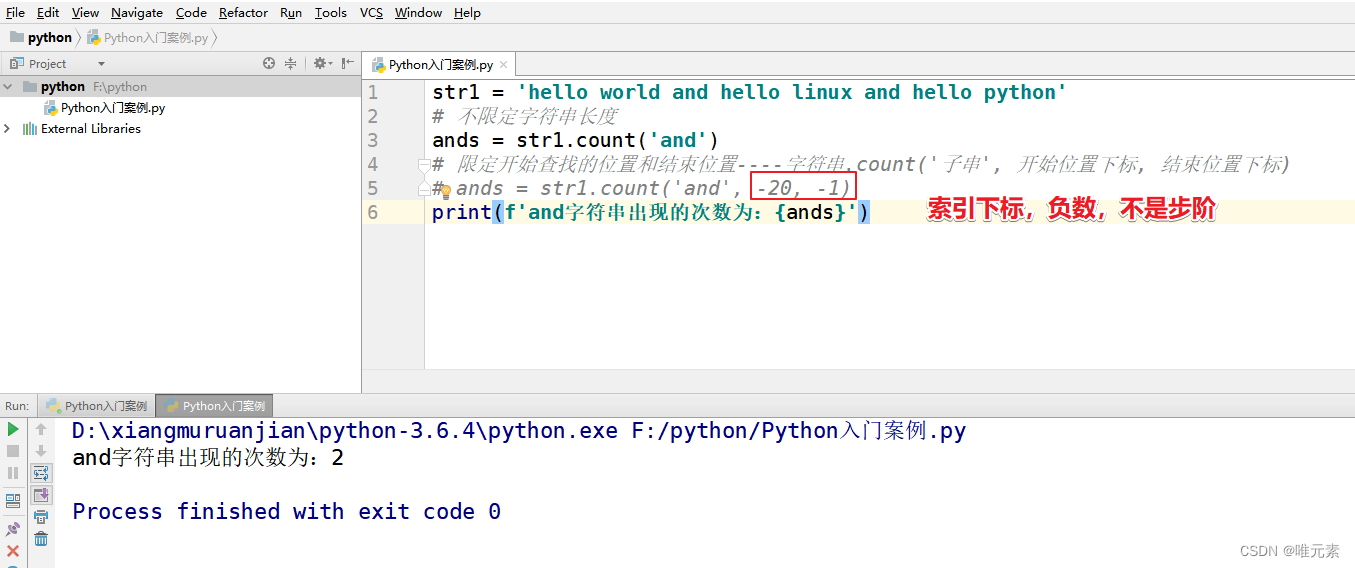
# 1. Python字符串处理概述**
字符串处理是Python中至关重要的任务,它提供了广泛的方法来操作和分析文本数据。字符串本质上是不可变的,这意味着一旦创建,就不能直接修改其内容。然而,我们可以使用各种函数和技术来创建新的字符串或修改现有字符串。
Python提供了一系列内置函数,如`len()`和`count()`,用于执行基本字符串操作。此外,正则表达式模块提供了强大的模式匹配功能,可用于复杂字符串处理任务。通过理解这些工具和技术,我们可以有效地处理和分析文本数据,从而解决各种实际问题。
# 2. 字符串计数技巧
### 2.1 内置函数计数
#### 2.1.1 count()
`count()` 函数用于计算字符串中指定子字符串出现的次数。其语法如下:
```python
str.count(substring, start=0, end=len(str))
```
* **参数说明:**
* `substring`: 要查找的子字符串。
* `start`: 开始搜索的位置(默认为 0)。
* `end`: 停止搜索的位置(默认为字符串长度)。
#### 代码示例:
```python
text = "Hello, world! Hello, Python!"
# 统计字符串中 "Hello" 出现的次数
count = text.count("Hello")
print(count) # 输出:2
```
#### 2.1.2 find()
`find()` 函数用于查找字符串中指定子字符串的第一个出现位置。其语法如下:
```python
str.find(substring, start=0, end=len(str))
```
* **参数说明:**
* `substring`: 要查找的子字符串。
* `start`: 开始搜索的位置(默认为 0)。
* `end`: 停止搜索的位置(默认为字符串长度)。
#### 代码示例:
```python
text = "Hello, world! Hello, Python!"
# 查找字符串中 "Hello" 的第一个出现位置
index = text.find("Hello")
print(index) # 输出:0
```
### 2.2 正则表达式计数
#### 2.2.1 re.findall()
`re.findall()` 函数用于查找字符串中所有匹配正则表达式的子字符串。其语法如下:
```python
re.findall(pattern, string)
```
* **参数说明:**
* `pattern`: 正则表达式模式。
* `string`: 要搜索的字符串。
#### 代码示例:
```python
import re
text = "Hello, world! Hello, Python!"
# 统计字符串中所有数字出现的次数
count = len(re.findall(r"\d+", text))
print(count) # 输出:2
```
#### 2.2.2 re.search()
`re.search()` 函数用于查找字符串中第一个匹配正则表达式的子字符串。其语法如下:
```python
re.search(pattern, string)
```
* **参数说明:**
* `pattern`: 正则表达式模式。
* `string`: 要搜索的字符串。
#### 代码示例:
```python
import re
text = "Hello, world! Hello, Python!"
# 查找字符串中第一个以 "H" 开头的单词
match = re.search(r"H\w+", text)
if match:
print(match.group()) # 输出:Hello
```
### 2.3 循环计数
#### 2.3.1 for 循环
for 循环可以遍历字符串中的每个字符,并计数特定字符出现的次数。其语法如下:
```python
for char in string:
# 对每个字符进行操作
```
#### 代码示例:
```python
text = "Hello, world!"
# 统计字符串中字母 "o" 出现的次数
count = 0
for char in text:
if char == "o":
count += 1
print(count) # 输出:2
```
#### 2.3.2 while 循环
while 循环也可以遍历字符串中的每个字符,并计数特定字符出现的次数。其语法如下:
```python
index = 0
while index < len(string):
# 对每个字符进行操作
index += 1
```
#### 代码示例:
```python
text = "Hello, world!"
# 统计字符串中字母 "o" 出现的次数
count = 0
index = 0
while index < len(text):
if text[index] == "o":
count += 1
index += 1
print(count) # 输出:2
```
# 3.1 忽略大小写计数
在某些情况下,我们可能需要忽略字符串中的大小写差异来进行计数。Python 提供了内置函数 `lower()` 和 `upper()` 来将字符串转换为小写或大写。
#### 3.1.1 lower()
`lower()` 函数将字符串中的所有字符转换为小写。它不会修改原始字符串,而是返回一个新的字符串。
```python
>>> text = "Hello World"
>>> text_lower = text.lower()
>>> print(text_lower)
hello world
```
#### 3.1.2 upper()
`upper()` 函数将字符串中的所有字符转换为大写。它也不会修改原始字符串,而是返回一个新的字符串。
```python
>>> text = "Hello World"
>>> text_upper = text.upper()
>>> print(text_upper)
HELLO WORLD
```
使用 `lower()` 或 `upper()` 函数可以将字符串转换为统一的大小写,从而忽略大小写差异进行计数。
### 3.2 多个字符计数
有时,我们需要同时计数多个字符。正则表达式提供了 `re.findall()` 和 `re.search()` 函数来匹配和计数多个字符。
#### 3.2.1 re.findall()
`re.findall()` 函数返回一个包含所有匹配子串的列表。它可以指定多个字符作为参数。
```python
>>> import re
>>> text = "Hello World, this is a test"
>>> matches = re.findall("[aeiou]", text)
>>> print(matches)
['e', 'o', 'i', 'a', 'e', 'i', 'o', 'a']
```
#### 3.2.2 re.search()
`re.search()` 函数返回第一个匹配子串的对象。它也可以指定多个字符作为参数。
```python
>>> import re
>>> text = "Hello World, this is a test"
>>> match = re.search("[aeiou]", text)
>>> print(match.group())
e
```
### 3.3 计数特定字符范围
正则表达式还允许我们指定字符范围。这可以用来计数特定范围内的字符。
#### 3.3.1 re.findall()
`re.findall()` 函数可以使用字符范围作为参数。
```python
>>> import re
>>> text = "Hello World, this is a test"
>>> matches = re.findall("[a-z]", text)
>>> print(matches)
['h', 'e', 'l', 'l', 'o', 'w', 'o', 'r', 'l', 'd', 't', 'h', 'i', 's', 'i', 's', 'a', 't', 'e', 's', 't']
```
#### 3.3.2 re.search()
`re.search()` 函数也可以使用字符范围作为参数。
```python
>>> import re
>>> text = "Hello World, this is a test"
>>> match = re.search("[a-z]", text)
>>> print(match.group())
h
```
# 4. 字符串计数应用
### 4.1 文本分析
#### 4.1.1 统计单词频率
字符串计数在文本分析中非常有用,例如统计单词频率。这对于自然语言处理、信息检索和文本挖掘等应用至关重要。
```python
import string
import nltk
# 准备文本数据
text = "This is a sample text for word frequency analysis. This text contains multiple words and can be used to demonstrate the counting of words."
# 转换文本为小写并删除标点符号
text = text.lower()
text = text.translate(str.maketrans('', '', string.punctuation))
# 使用 NLTK 库分词
words = nltk.word_tokenize(text)
# 创建一个字典来存储单词频率
word_freq = {}
for word in words:
if word not in word_freq:
word_freq[word] = 0
word_freq[word] += 1
# 打印单词频率
for word, freq in word_freq.items():
print(f"{word}: {freq}")
```
**代码逻辑分析:**
* 将文本转换为小写并删除标点符号,以标准化单词。
* 使用 NLTK 库分词,将文本分解为单词列表。
* 创建一个字典来存储单词频率,如果单词不存在,则初始化为 0。
* 遍历单词列表,并为每个单词增加频率。
* 打印单词及其频率。
#### 4.1.2 检测重复字符
字符串计数还可以用于检测重复字符,这对于数据清理和异常检测很有用。
```python
# 定义一个字符串
string = "abcabcbb"
# 创建一个字典来存储字符频率
char_freq = {}
for char in string:
if char not in char_freq:
char_freq[char] = 0
char_freq[char] += 1
# 检查是否有重复字符
has_duplicates = False
for char, freq in char_freq.items():
if freq > 1:
has_duplicates = True
break
# 打印结果
if has_duplicates:
print("字符串中存在重复字符。")
else:
print("字符串中没有重复字符。")
```
**代码逻辑分析:**
* 创建一个字典来存储字符频率,如果字符不存在,则初始化为 0。
* 遍历字符串,并为每个字符增加频率。
* 检查字典中是否有频率大于 1 的字符,如果有,则表明存在重复字符。
* 打印结果。
### 4.2 数据验证
#### 4.2.1 验证电子邮件地址
字符串计数在数据验证中也很重要,例如验证电子邮件地址。
```python
import re
# 定义电子邮件地址正则表达式
email_regex = r"^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$"
# 定义要验证的电子邮件地址
email = "example@domain.com"
# 使用正则表达式匹配电子邮件地址
match = re.match(email_regex, email)
# 检查匹配结果
if match:
print("电子邮件地址有效。")
else:
print("电子邮件地址无效。")
```
**代码逻辑分析:**
* 定义一个正则表达式来匹配有效的电子邮件地址。
* 使用正则表达式匹配给定的电子邮件地址。
* 检查匹配结果,如果匹配,则电子邮件地址有效,否则无效。
#### 4.2.2 验证密码强度
字符串计数还可以用于验证密码强度。
```python
import string
# 定义密码强度规则
min_length = 8
min_uppercase = 1
min_lowercase = 1
min_digits = 1
min_special = 1
# 定义要验证的密码
password = "Password123!"
# 检查密码长度
if len(password) < min_length:
print("密码太短。")
# 检查密码中大写字母的数量
uppercase_count = 0
for char in password:
if char.isupper():
uppercase_count += 1
if uppercase_count < min_uppercase:
print("密码中大写字母太少。")
# 检查密码中小写字母的数量
lowercase_count = 0
for char in password:
if char.islower():
lowercase_count += 1
if lowercase_count < min_lowercase:
print("密码中小写字母太少。")
# 检查密码中数字的数量
digit_count = 0
for char in password:
if char.isdigit():
digit_count += 1
if digit_count < min_digits:
print("密码中数字太少。")
# 检查密码中特殊字符的数量
special_count = 0
for char in password:
if char in string.punctuation:
special_count += 1
if special_count < min_special:
print("密码中特殊字符太少。")
# 检查密码强度
if uppercase_count >= min_uppercase and lowercase_count >= min_lowercase and digit_count >= min_digits and special_count >= min_special:
print("密码强度强。")
else:
print("密码强度弱。")
```
**代码逻辑分析:**
* 定义密码强度规则,包括最小长度、大写字母数量、小写字母数量、数字数量和特殊字符数量。
* 检查密码是否满足每个规则。
* 如果密码满足所有规则,则密码强度强,否则密码强度弱。
# 5. 字符串计数最佳实践
### 5.1 性能优化
**5.1.1 使用内置函数**
在可能的情况下,应优先使用内置函数来进行字符串计数。内置函数通常经过高度优化,可以比自定义循环或正则表达式更快地执行。
**示例:**
```python
# 使用 count() 函数计数字符
string = "Hello, world!"
char_count = string.count("l")
# 使用 find() 函数查找字符并计数
string = "Hello, world!"
char_count = string.find("l") + 1
```
### 5.1.2 避免不必要的循环
循环对于字符串计数很有用,但如果使用不当,可能会导致性能问题。避免在不必要的情况下使用循环,例如当可以使用内置函数或正则表达式时。
**示例:**
```python
# 避免使用循环来计数字符
string = "Hello, world!"
char_count = 0
for char in string:
if char == "l":
char_count += 1
# 使用 count() 函数来计数字符
string = "Hello, world!"
char_count = string.count("l")
```
### 5.2 可读性和可维护性
**5.2.1 使用描述性变量名**
使用描述性的变量名可以提高代码的可读性和可维护性。避免使用晦涩或不直观的变量名,例如 `x` 或 `y`。
**示例:**
```python
# 使用描述性变量名
string = "Hello, world!"
char_count = string.count("l")
# 使用不直观的变量名
string = "Hello, world!"
x = string.count("l")
```
### 5.2.2 注释代码
注释代码可以解释代码的目的和功能,提高可读性和可维护性。使用清晰简洁的注释,避免使用技术术语或行话。
**示例:**
```python
# 使用注释解释代码
string = "Hello, world!"
# 计数字符串中字符 "l" 的出现次数
char_count = string.count("l")
```
### 5.3 异常处理
**5.3.1 处理输入错误**
在进行字符串计数之前,应验证输入是否有效。如果输入无效,则应引发异常或返回错误消息。
**示例:**
```python
try:
string = input("请输入字符串:")
char_count = string.count("l")
except ValueError:
print("输入无效,请输入字符串。")
```
**5.3.2 处理正则表达式错误**
在使用正则表达式进行字符串计数时,应处理潜在的正则表达式错误。如果正则表达式无效或输入字符串不匹配正则表达式,则应引发异常或返回错误消息。
**示例:**
```python
try:
pattern = r"[a-z]+"
matches = re.findall(pattern, string)
char_count = len(matches)
except re.error:
print("正则表达式无效,请检查正则表达式。")
```
# 6. 总结
本文全面探讨了 Python 中的字符串计数技术,从内置函数到正则表达式,再到循环计数。我们深入研究了高级计数方法,包括忽略大小写、计数多个字符和特定字符范围。
我们还讨论了字符串计数的实际应用,例如文本分析、数据验证和字符串加密。此外,我们强调了最佳实践,包括性能优化、可读性和异常处理。
通过掌握这些技术,Python 开发人员可以有效地处理字符串数据,从简单的字符计数到复杂的文本分析任务。
0
0
相关推荐
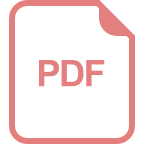
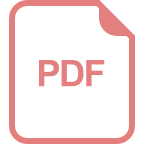
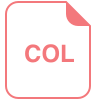
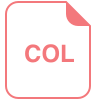
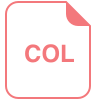
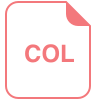
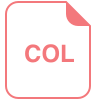
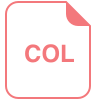