C#中 利用OpenCvSharp4 实现图片的旋转
时间: 2024-10-04 10:03:58 浏览: 16
在C#中,利用OpenCVSharp4库实现图片旋转,可以按照以下步骤进行:
首先,你需要安装OpenCVSharp4的NuGet包。在Visual Studio项目中右键点击解决方案 -> 管理NuGet程序 -> 搜索OpenCvSharp4并安装。
```csharp
using OpenCvSharp;
```
接下来,你可以创建一个方法来读取图片,并对其进行旋转。假设我们想将图像顺时针旋转90度:
```csharp
public Mat RotateImage(string imagePath)
{
// 读取原始图像
Mat originalImage = Cv2.ImRead(imagePath);
// 获取图像尺寸
int width = originalImage.Width;
int height = originalImage.Height;
// 创建一个新的旋转矩阵
Mat rotationMatrix = new Mat();
Cv2.GetRotationMatrix2D(new Point(width / 2, height / 2), -90, 1.0, out rotationMatrix);
// 计算旋转后的图像大小
Size rotatedSize = new Size(height, width); // 注意这里宽度变成了高度,反之亦然
// 执行旋转操作
Mat rotatedImage = new Mat(rotatedSize, originalImage.Type);
Cv2.WarpAffine(originalImage, rotatedImage, rotationMatrix, rotatedSize, InterpolationTypes.Bilinear, BorderMode.Constant, new Scalar(0));
return rotatedImage;
}
```
在这个例子中,`rotationMatrix`是一个二维变换矩阵,通过`WarpAffine`函数应用到原始图像上。`-90`表示逆时针旋转90度,正值则代表顺时针旋转。
相关推荐
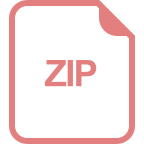
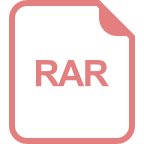
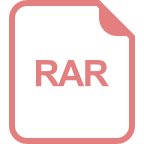
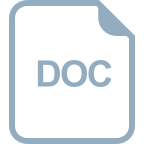


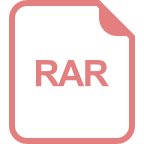
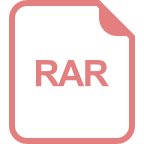
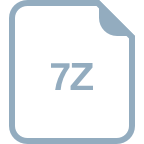
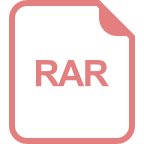
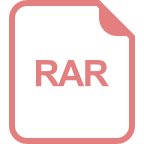
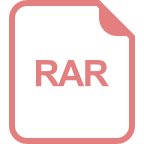
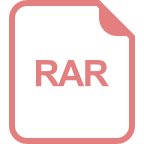
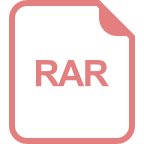
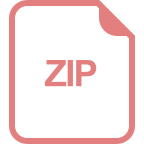
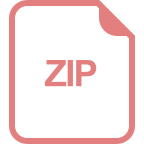
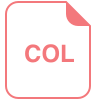