Python实现继承和多态
时间: 2023-11-05 12:57:54 浏览: 36
Python中的继承可以通过在子类中指定父类来实现。例如:
```python
class Animal:
def __init__(self, name):
self.name = name
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "汪汪汪"
class Cat(Animal):
def sound(self):
return "喵喵喵"
dog = Dog("小狗")
cat = Cat("小猫")
print(dog.sound()) # 输出:汪汪汪
print(cat.sound()) # 输出:喵喵喵
```
Python中的多态性可以通过方法重写来实现。例如:
```python
class Animal:
def __init__(self, name):
self.name = name
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "汪汪汪"
class Cat(Animal):
def sound(self):
return "喵喵喵"
def make_sound(animal):
print(animal.sound())
dog = Dog("小狗")
cat = Cat("小猫")
make_sound(dog) # 输出:汪汪汪
make_sound(cat) # 输出:喵喵喵
```
在这个例子中,`make_sound` 函数接受一个 `Animal` 类型的参数,但是由于 `Dog` 和 `Cat` 类都继承自 `Animal` 类并重写了 `sound` 方法,所以可以传入 `dog` 和 `cat` 对象来调用 `sound` 方法。这就体现了多态性。
相关推荐
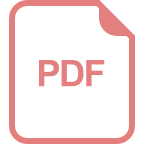
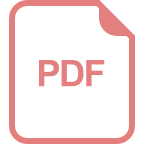














