帮我使用pytorch和opencv实现根据双目视差图生成点云
时间: 2023-02-20 08:51:23 浏览: 89
可以使用OpenCV库读取双目图像,并使用SGBM算法或BM算法计算视差图。然后,可以使用OpenCV的reprojectImageTo3D函数将视差图映射到三维空间中,生成点云。
以下是代码示例:
```
import cv2
import numpy as np
import torch
# 读入双目图像
imgL = cv2.imread('left.jpg', 0)
imgR = cv2.imread('right.jpg', 0)
# 计算视差图
stereo = cv2.createStereoBM(numDisparities=16, blockSize=15)
disparity = stereo.compute(imgL, imgR)
# 将视差图映射到三维空间
points = cv2.reprojectImageTo3D(disparity, Q)
# 将点云转换为pytorch tensor
points = torch.from_numpy(points).float()
```
其中,Q是用于重投影的投影矩阵,可以在双目相机标定过程中计算出。
相关问题
pytorch和opencv读取图片
PyTorch和OpenCV都是常用的图像处理库,它们都可以用来读取和处理图像数据。但是它们读取图像的方式略有不同。
PyTorch读取图像数据通常使用`loaddata`等函数实现,读取的数据格式为(C, H, W),即(通道数,高,宽)。为了将其转换成OpenCV格式,需要先将其转换成numpy通用的格式,再将其转换成OpenCV格式。
而OpenCV读取图像数据则直接使用`cv2.imread()`函数实现,读取的数据格式为OpenCV格式,即BGR格式的numpy数组。
需要注意的是,PyTorch和OpenCV读取图像的通道顺序不同,PyTorch的通道顺序为RGB,而OpenCV的通道顺序为BGR。因此,在将PyTorch格式的图像转换成OpenCV格式时,需要将通道顺序进行调整。
pytorch和opencv一起使用
可以将PyTorch与OpenCV一起使用,例如使用OpenCV读取图像并将其转换为PyTorch的张量格式,然后进行深度学习模型的训练和预测等操作。也可以在PyTorch中使用OpenCV中的函数对图像进行处理,如图像增强、裁剪、旋转等操作。
相关推荐
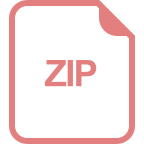
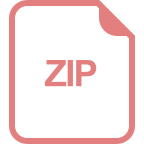
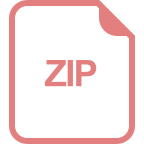
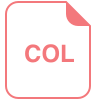
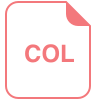

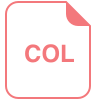
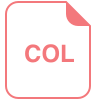







