stm32控制ws2812灯带,要求产生三种不同亮度的灯光,用stm32标准库编程完成,标准库
时间: 2023-08-22 17:04:20 浏览: 125
实现串行通信,向灯带发送控制信号,控制灯光的亮度。
以下是大致的程序框架:
1. 定义灯带控制端口和引脚,初始化端口。
2. 定义发送函数,将控制信号通过串行通信发送到灯带。
3. 定义控制函数,根据不同的亮度,生成控制信号,调用发送函数发送给灯带。
具体实现步骤如下:
1. 定义控制端口和引脚。
```C
#define LED_PORT GPIOA
#define LED_PIN GPIO_Pin_5
```
2. 初始化端口。
```C
GPIO_InitTypeDef GPIO_InitStructure;
// 打开GPIO和定时器时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
```
3. 定义发送函数。
```C
void send_byte(uint8_t data)
{
uint8_t i;
for (i = 0; i < 8; i++)
{
if ((data & 0x80) == 0x80)
{
LED_PORT->BSRRL = LED_PIN;
__NOP(); __NOP(); __NOP();
__NOP(); __NOP(); __NOP();
LED_PORT->BSRRH = LED_PIN;
__NOP(); __NOP(); __NOP();
}
else
{
LED_PORT->BSRRL = LED_PIN;
__NOP(); __NOP(); __NOP();
LED_PORT->BSRRH = LED_PIN;
__NOP(); __NOP(); __NOP();
__NOP(); __NOP(); __NOP();
}
data <<= 1;
}
}
```
4. 定义控制函数,根据不同的亮度生成控制信号。
```C
void set_light(uint8_t level)
{
uint8_t i;
for (i = 0; i < 24; i++)
{
if (i % 8 == 0)
{
send_byte(0);
}
else if (i < level)
{
send_byte(0xE0);
}
else
{
send_byte(0x80);
}
}
}
```
5. 在主函数中调用控制函数,可以产生不同亮度的灯光。
```C
int main(void)
{
uint32_t i;
// 初始化端口
init_led();
// 控制灯光亮度
while (1)
{
for (i = 0; i < 1000000; i++);
set_light(8);
for (i = 0; i < 1000000; i++);
set_light(16);
for (i = 0; i < 1000000; i++);
set_light(24);
}
}
```
这样就可以实现控制ws2812灯带产生三种不同亮度的灯光了。
阅读全文
相关推荐
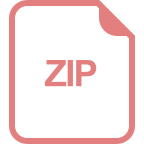
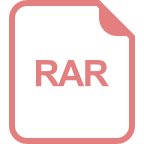



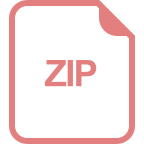
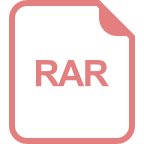
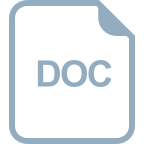
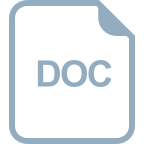
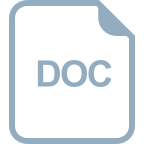
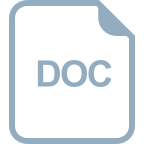




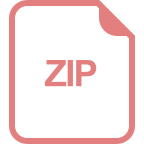
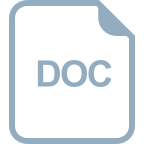
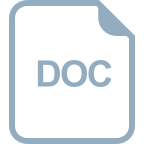