抽取doc格式文件中含有关键词的句子,并将这些句子自动整理存储到新的DOC格式文件中,python代码多线程实现
时间: 2023-09-20 11:05:56 浏览: 179
### 回答1:
您可以使用 Python 中的多线程来实现这一功能。您需要使用 Python 的文件操作模块来读取原始文件,再使用正则表达式来匹配文本中包含关键词的句子。
这里是一个基本的实现思路:
1. 使用 Python 的文件操作模块读取原始文件。
2. 使用正则表达式匹配文本中包含关键词的句子。
3. 将匹配到的句子存储到新的 DOC 格式文件中。
4. 使用 Python 的多线程模块创建多个线程来同时处理文件。
下面是一个简单的例子,它使用了 Python 的多线程模块 `threading` 和文件操作模块 `io` 来实现上述功能:
```
import threading
import io
import re
# 读取原始文件
with io.open('original.doc', 'r', encoding='utf-8') as f:
text = f.read()
# 使用正则表达式匹配文本中包含关键词的句子
pattern = re.compile(r'关键词')
sentences = pattern.findall(text)
# 将匹配到的句子存储到新的 DOC 格式文件中
with io.open('output.doc', 'w', encoding='utf-8') as f:
for sentence in sentences:
f.write(sentence + '\n')
# 使用多线程处理文件
def process_file(filename):
# 处理文件的代码
threads = []
for filename in ['file1.doc', 'file2.doc', 'file3.doc']:
t = threading.Thread(target=process_file, args=(filename,))
threads.append(t)
t.start()
for t in threads:
t.join()
print('Done!')
```
### 回答2:
抽取doc格式文件中含有关键词的句子,并将这些句子自动整理存储到新的DOC格式文件中,可以使用Python的多线程来实现。
首先,需要安装Python的docx库,该库可以用来读取和写入doc格式文件。可以使用以下命令来安装该库:
```
pip install python-docx
```
接下来,编写Python代码实现任务的自动化。代码的基本思路如下:
1. 打开原始的doc文件,通过docx库读取文件内容;
2. 通过输入关键词,检查每个句子是否包含关键词;
3. 如果句子包含关键词,则将该句子添加到一个列表中;
4. 创建一个新的doc文件,通过docx库写入列表中的句子;
5. 使用多线程来实现并发处理。
下面是一个示例代码的框架:
```python
import docx
import multiprocessing as mp
# 输入原始doc文件的路径
original_file_path = "original.docx"
# 输入新的doc文件路径
new_file_path = "new.docx"
# 输入关键词
keyword = "关键词"
# 读取原始文档
def read_original_file():
doc = docx.Document(original_file_path)
sentences = []
for paragraph in doc.paragraphs:
for sentence in paragraph.text.split("。"):
sentences.append(sentence.strip())
return sentences
# 写入新的文档
def write_new_file(sentences):
doc = docx.Document()
for sentence in sentences:
doc.add_paragraph(sentence)
doc.save(new_file_path)
# 处理句子
def process_sentence(sentence):
# 判断句子是否包含关键词
if keyword in sentence:
return sentence
# 多线程处理
def multi_thread_processing(sentences):
pool = mp.Pool(mp.cpu_count())
results = pool.map(process_sentence, sentences)
pool.close()
pool.join()
return list(filter(None, results))
# 主函数
def main():
# 读取原始文档
sentences = read_original_file()
# 多线程处理句子
processed_sentences = multi_thread_processing(sentences)
# 写入新的文档
write_new_file(processed_sentences)
if __name__ == "__main__":
main()
```
以上代码实现了对doc文件的读取、过滤句子、并发处理以及写入新的doc文件的功能。可以根据实际需求进行修改和优化。
### 回答3:
要使用Python实现抽取DOC格式文件中含有关键词的句子并存储到新的DOC文件中,可以使用python-docx库来读写DOC文件,并使用多线程来提高处理效率。以下是一个可能的实现:
```python
import docx
import threading
# 定义关键词
keywords = ["关键词1", "关键词2", "关键词3"]
# 创建一个新的DOC文件用于存储匹配到的句子
output_doc = docx.Document()
# 加载原始DOC文件
input_doc = docx.Document("原始文件.docx")
# 锁,用于保护输出文件写入操作
lock = threading.Lock()
# 处理每个段落的函数
def process_paragraph(paragraph):
# 遍历段落中的每个句子
for sentence in paragraph.sentences:
# 判断句子是否包含关键词
if any(keyword in sentence.text for keyword in keywords):
# 使用锁保护写入操作
with lock:
# 将匹配到的句子写入到输出文件中
output_doc.add_paragraph(sentence.text)
# 处理每个段落的线程函数
def process_thread(paragraphs):
for paragraph in paragraphs:
process_paragraph(paragraph)
# 创建线程列表
threads = []
# 将段落列表平均分配给每个线程
num_threads = 4
chunk_size = len(input_doc.paragraphs) // num_threads
for i in range(num_threads):
start_idx = i * chunk_size
end_idx = start_idx + chunk_size
thread = threading.Thread(target=process_thread, args=(input_doc.paragraphs[start_idx:end_idx],))
threads.append(thread)
thread.start()
# 等待所有线程完成
for thread in threads:
thread.join()
# 保存输出文件
output_doc.save("新文件.docx")
```
这段代码使用了`docx`库来读取和写入DOC文件。首先,我们定义了关键词列表。然后,我们创建了一个新的DOC文件对象 `output_doc`,用于存储匹配到的句子。接着,我们加载原始DOC文件,创建一个锁对象 `lock`,用于保护写入操作。然后,我们定义了一个处理每个段落的函数 `process_paragraph`,遍历每个句子并检查是否包含关键词,若匹配则写入到 `output_doc` 中。接下来,我们定义了一个处理每个段落的线程函数 `process_thread`,将每个段落列表平均分配给每个线程进行处理。然后,我们创建了多个线程对象,并通过调用 `start()` 方法来启动线程。最后,我们等待所有线程完成,并保存输出文件 `output_doc`。
阅读全文
相关推荐
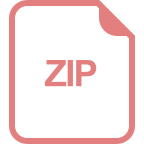
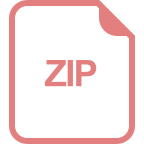
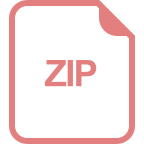
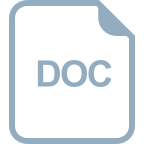
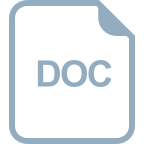
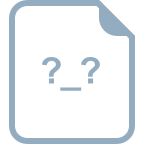
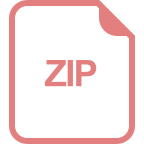
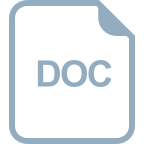
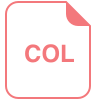
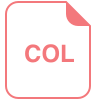
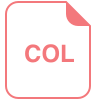
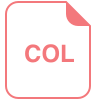
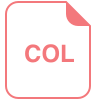
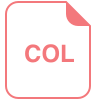
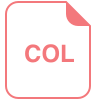
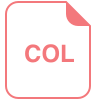
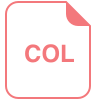
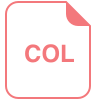
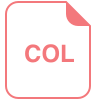