函数实现getline
时间: 2023-05-16 10:04:44 浏览: 97
函数实现getline可以使用C语言中的fgets函数,示例代码如下:
char* getline(char* str, int size) {
char* result = fgets(str, size, stdin);
if (result != NULL) {
int len = strlen(str);
if (str[len - 1] == '\n') {
str[len - 1] = '\0';
}
}
return result;
}
这个函数可以读取用户从标准输入中输入的一行字符串,并去掉行末的换行符。
相关问题
c语言函数实现getline
C语言并没有标准的getline函数,但可以通过自定义函数来实现类似功能。以下是一个简单的例子:
```
#include <stdio.h>
#include <stdlib.h>
char *getline() {
char *line = NULL;
int bufsize = 0;
int i = 0;
char c;
do {
c = getchar();
if (i >= bufsize) {
bufsize += 10;
line = realloc(line, bufsize);
}
line[i++] = c;
} while(c != '\n' && c != EOF);
line[--i] = '\0'; // remove newline or EOF character
return line;
}
int main() {
char *line;
printf("Enter a line: ");
line = getline();
printf("You entered: %s\n", line);
free(line);
return 0;
}
```
这个函数读取标准输入中的一行,并将其作为字符串返回。函数中使用了动态内存分配(realloc)来根据行的长度动态调整字符串的大小。函数执行结束后,需要手动释放字符串的内存空间。
C++实现getline函数
getline函数可以用于从标准输入或文件中读取一行数据,并将其存储在一个字符数组中。以下是一个简单的C语言实现getline函数的代码:
```
#include <stdio.h>
#include <stdlib.h>
char *getline(void) {
char *line = NULL;
size_t bufsize = 0;
getline(&line, &bufsize, stdin);
return line;
}
int main() {
char *line = getline();
printf("You entered: %s", line);
free(line);
return 0;
}
```
这个实现使用了标准库函数getline来读取输入,它会自动分配足够的内存来存储输入行。在函数返回时,需要手动释放分配的内存。
阅读全文
相关推荐
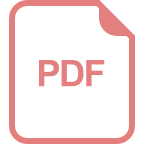













