C# 读取csv文件某一列的数据
时间: 2023-08-13 17:07:40 浏览: 707
你可以使用 C# 中的 StreamReader 和 CsvHelper 库来读取 CSV 文件的某一列数据。以下是一个简单的示例代码:
```csharp
using System;
using System.IO;
using CsvHelper;
class Program
{
static void Main(string[] args)
{
using (var reader = new StreamReader("file.csv"))
using (var csv = new CsvReader(reader))
{
// 读取 CSV 文件的全部数据
var records = csv.GetRecords<dynamic>();
// 读取某一列的数据
foreach (var record in records)
{
Console.WriteLine(record.ColumnName);
}
}
}
}
```
在这个示例中,我们使用 CsvHelper 库来读取 CSV 文件的全部数据,然后在 foreach 循环中输出了某一列的数据。你需要将代码中的 "file.csv" 替换为你要读取的文件路径,"ColumnName" 替换为你要读取的列名。
相关问题
C#读取csv文件,某一列数据出现的数字
在C#中,可以使用`System.IO`和`System.Data`命名空间下的类库来读取CSV文件。如果你想要获取CSV文件中某一列的数字,通常会使用`StreamReader`读取文件行,然后通过字符串处理提取出所需的列。这里是一个简单的示例:
```csharp
using System;
using System.IO;
using System.Linq;
class Program
{
static void Main(string[] args)
{
string filePath = "path_to_your_csv_file.csv"; // 替换为你的CSV文件路径
string delimiter = ",";
try
{
using (var reader = new StreamReader(filePath))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture, delimiter))
{
var records = csv.GetRecords<dynamic>(); // 使用dynamic类型方便动态访问属性
int[] columnNumbers = Enumerable.Range(0, records.Count()).Where((_, index) => IsNumber(records[index].YourColumnColumnName)).ToArray(); // 把你想检查的列名替换为"YourColumnColumnName"
if (columnNumbers.Any())
{
Console.WriteLine($"Column contains numbers: {string.Join(", ", columnNumbers)}");
}
else
{
Console.WriteLine("No numeric values found in the specified column.");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error reading CSV file: {ex.Message}");
}
}
static bool IsNumber(object value)
{
return decimal.TryParse(value?.ToString(), out _); // 如果值能转换为decimal,则认为是数字
}
}
```
在这个例子中,你需要将`filePath`变量替换为你要读取的实际CSV文件路径,以及在`IsNumber`函数中将`YourColumnColumnName`替换为你想检查的列的名称。这个脚本会找出指定列中有多少数字,并打印出来。
C# 读取csv文件时,某一列包含json数据该如何处理?
在C#中读取CSV文件时,如果某一列的数据实际上是JSON格式的,你需要先将这一列解析成JSON对象。这通常涉及到以下几个步骤:
1. **导入所需库**:首先需要添加`System.Text.Json`命名空间,它包含了JSON的处理功能。
```csharp
using System.IO;
using System.Text.Json;
```
2. **打开CSV文件**:使用`StreamReader`或`File`类打开CSV文件并逐行读取。
```csharp
string csvFilePath = "path_to_your_file.csv";
string line;
List<Dictionary<string, dynamic>> data = new List<Dictionary<string, dynamic>>();
using (var reader = new StreamReader(csvFilePath))
{
while ((line = reader.ReadLine()) != null)
{
//...
}
}
```
3. **解析JSON列**:当你遇到包含JSON的那列,会跳过其他字段并将该行转换为字符串,然后尝试解析为JsonDocument或JsonObject。
```csharp
if (!string.IsNullOrEmpty(line) && line.Contains("{"))
{
string jsonColumn = line.Split(',')[columnIndex]; //假设columnIndex是你JSON列的位置
JsonElement jsonObject;
try
{
jsonObject = JsonDocument.Parse(jsonColumn);
//...
}
catch (Exception e)
{
//处理JSON解析错误
Console.WriteLine($"Error parsing JSON: {e.Message}");
}
}
//将解析后的Json对象添加到数据字典中
data.Add(new Dictionary<string, object> { { "json_column", jsonObject } });
```
4. **存储结果**:最后,你可以选择将这些字典存入列表中,以便后续操作或转换为更合适的模型。
阅读全文
相关推荐
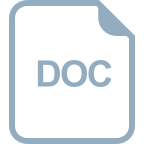
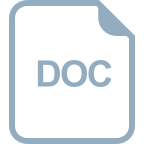
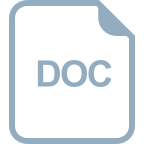

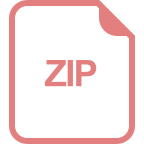
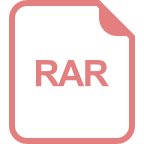
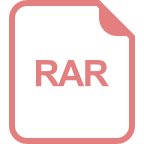
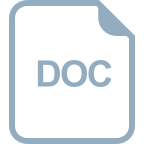
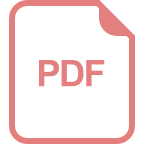
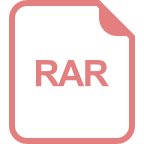






