c++实现string到enum的转换
时间: 2024-04-29 17:21:26 浏览: 15
假设我们有一个枚举类型:
```c++
enum Color {
RED,
GREEN,
BLUE
};
```
以及一个字符串:
```c++
std::string colorStr = "GREEN";
```
我们可以实现一个函数,将字符串转换为对应的枚举值:
```c++
Color strToColor(const std::string& colorStr) {
if (colorStr == "RED") {
return RED;
} else if (colorStr == "GREEN") {
return GREEN;
} else if (colorStr == "BLUE") {
return BLUE;
} else {
// 处理无法转换的情况
throw std::runtime_error("Invalid color string");
}
}
```
使用方法:
```c++
Color color = strToColor(colorStr);
```
同样,我们也可以实现一个将枚举值转换为字符串的函数:
```c++
std::string colorToStr(Color color) {
switch (color) {
case RED:
return "RED";
case GREEN:
return "GREEN";
case BLUE:
return "BLUE";
default:
// 处理无法转换的情况
throw std::runtime_error("Invalid color value");
}
}
```
使用方法:
```c++
std::string colorStr = colorToStr(color);
```
相关推荐
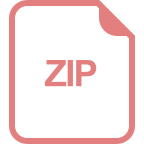
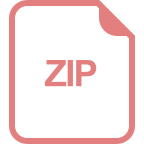
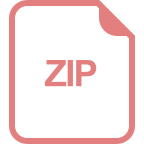














