opencv 图像轮廓匹配中轮廓筛选c++代码实现
时间: 2023-11-13 08:03:01 浏览: 137
以下是基于OpenCV的C++代码实现图像轮廓匹配中的轮廓筛选:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取模板图像和目标图像
Mat templateImg = imread("template.jpg", IMREAD_GRAYSCALE);
Mat targetImg = imread("target.jpg", IMREAD_GRAYSCALE);
// 定义轮廓存储变量
vector<vector<Point>> templateContours, targetContours;
vector<Vec4i> templateHierarchy, targetHierarchy;
// 寻找模板图像和目标图像的轮廓
findContours(templateImg, templateContours, templateHierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
findContours(targetImg, targetContours, targetHierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 定义轮廓筛选阈值
double minArea = 100;
double maxArea = 1000;
// 对模板图像轮廓进行筛选
vector<vector<Point>> filteredTemplateContours;
for (int i = 0; i < templateContours.size(); i++)
{
double area = contourArea(templateContours[i]);
if (area > minArea && area < maxArea)
{
filteredTemplateContours.push_back(templateContours[i]);
}
}
// 对目标图像轮廓进行筛选
vector<vector<Point>> filteredTargetContours;
for (int i = 0; i < targetContours.size(); i++)
{
double area = contourArea(targetContours[i]);
if (area > minArea && area < maxArea)
{
filteredTargetContours.push_back(targetContours[i]);
}
}
// 显示筛选后的模板图像和目标图像轮廓
Mat templateContoursImg = Mat::zeros(templateImg.size(), CV_8UC3);
drawContours(templateContoursImg, filteredTemplateContours, -1, Scalar(0, 255, 0), 2);
imshow("Filtered Template Contours", templateContoursImg);
Mat targetContoursImg = Mat::zeros(targetImg.size(), CV_8UC3);
drawContours(targetContoursImg, filteredTargetContours, -1, Scalar(0, 255, 0), 2);
imshow("Filtered Target Contours", targetContoursImg);
waitKey(0);
return 0;
}
```
注:以上代码中的`template.jpg`和`target.jpg`分别为模板图像和目标图像,可以根据实际情况进行修改。
阅读全文
相关推荐
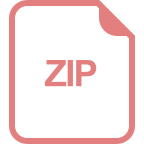
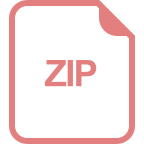
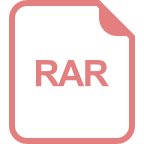
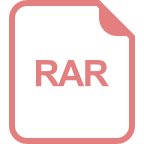
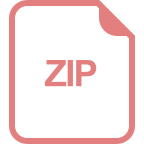
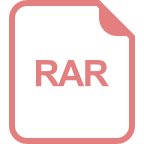
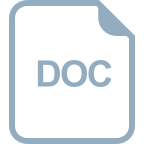
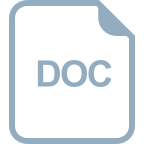
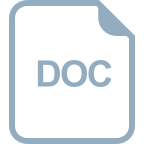
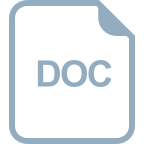
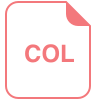
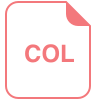
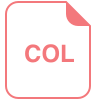
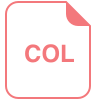
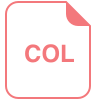
