@EqualsAndHashCode(callSuper = true
时间: 2023-10-20 19:32:00 浏览: 39
对于Java中的`@EqualsAndHashCode(callSuper = true)`注解,它用于生成`equals()`和`hashCode()`方法,以及继承自父类的字段。
当我们在一个子类中使用`@EqualsAndHashCode(callSuper = true)`注解时,它将会在生成的方法中包含父类的字段,以确保父类中定义的字段也被考虑在内。
这个注解可以很方便地在子类中实现对象的相等性比较和哈希码计算。
相关问题
@EqualsAndHashCode(callSuper = true) 红
@EqualsAndHashCode(callSuper = true) 是Lombok注解中的一个选项,用于在生成equals和hashCode方法时包含父类的属性。如果你在一个子类中使用了@Data注解,但没有使用@EqualsAndHashCode(callSuper = true),那么生成的equals和hashCode方法将只比较子类自身的属性,而不包括父类的属性。这可能会导致equals方法在比较两个不同子类对象时返回true,因为它们的自身属性相同,而不考虑父类的属性。
例如,假设有一个父类A,它有一个属性id,以及两个子类B和C,它们都继承自A,并且都有一个属性name。如果在B和C中使用了@Data注解,但没有使用@EqualsAndHashCode(callSuper = true),那么在比较B和C对象时,只会比较它们自身的name属性,而不考虑父类A的id属性。这可能导致错误的相等判断。
为了解决这个问题,可以在子类上添加@EqualsAndHashCode(callSuper = true),这样生成的equals和hashCode方法将包含父类的属性,从而正确比较两个不同子类对象的相等性。
@equalsandhashcode(callsuper = true)报错
当我们在自定义类中重写equals()和hashCode()方法时,通常需要调用父类的equals()和hashCode()方法来确保遵循Java的一致性规则。在使用注解@EqualsAndHashCode(callSuper = true)时,如果父类也重写了equals()和hashCode()方法,那么在生成子类的equals()和hashCode()方法时会调用父类的equals()和hashCode()方法,确保了对象的一致性。
然而,如果调用父类的equals()和hashCode()方法时报错,则可能有以下原因:
1. 父类没有重写Object类的equals()和hashCode()方法:如果使用了@EqualsAndHashCode(callSuper = true)注解,但是父类没有重写equals()和hashCode()方法,则会导致编译错误。此时,我们可以尝试在父类中重写equals()和hashCode()方法,或者在子类中使用自定义的equals()和hashCode()方法。
2. 父类的equals()和hashCode()方法的访问权限不允许子类访问:如果父类的equals()和hashCode()方法是private访问权限,那么子类是无法继承这些方法的,即使使用了@EqualsAndHashCode(callSuper = true)注解也不行。这种情况下,我们可以尝试修改父类的equals()和hashCode()方法的访问权限为protected或public。
总之,在使用@EqualsAndHashCode(callSuper = true)注解时,需要确保父类重写了equals()和hashCode()方法,并且子类可以正确访问父类的这两个方法,才能避免报错。
相关推荐
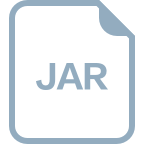
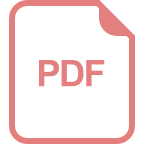
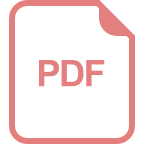












