帮我写一个web端RSA签名验签的Python代码
时间: 2023-08-28 08:12:07 浏览: 209
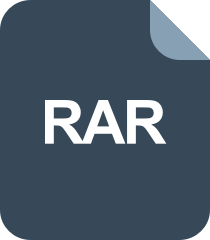
(纯算法,没有调用库)python实现RSA数字签名代码、ppt、文档.rar

好的,以下是一个简单的web端RSA签名验签的Python代码:
```python
import base64
import hashlib
from Crypto.PublicKey import RSA
from Crypto.Signature import PKCS1_v1_5
from Crypto.Cipher import PKCS1_v1_5 as Cipher_PKCS1_v1_5
from Crypto.Hash import SHA256
# 生成RSA公私钥对
def generate_key_pair():
key = RSA.generate(1024)
private_key = key.export_key()
public_key = key.publickey().export_key()
return private_key, public_key
# RSA签名
def rsa_sign(message, private_key):
key = RSA.import_key(private_key)
h = SHA256.new(message.encode())
signer = PKCS1_v1_5.new(key)
signature = signer.sign(h)
return base64.b64encode(signature).decode()
# RSA验签
def rsa_verify(message, signature, public_key):
key = RSA.import_key(public_key)
h = SHA256.new(message.encode())
verifier = PKCS1_v1_5.new(key)
signature = base64.b64decode(signature)
return verifier.verify(h, signature)
# RSA加密
def rsa_encrypt(message, public_key):
key = RSA.import_key(public_key)
cipher = Cipher_PKCS1_v1_5.new(key)
ciphertext = cipher.encrypt(message.encode())
return base64.b64encode(ciphertext).decode()
# RSA解密
def rsa_decrypt(ciphertext, private_key):
key = RSA.import_key(private_key)
cipher = Cipher_PKCS1_v1_5.new(key)
message = cipher.decrypt(base64.b64decode(ciphertext), None)
return message.decode()
# 测试代码
if __name__ == '__main__':
# 生成RSA公私钥对
private_key, public_key = generate_key_pair()
# 签名
message = 'hello world'
signature = rsa_sign(message, private_key)
print('signature:', signature)
# 验签
is_valid = rsa_verify(message, signature, public_key)
print('is_valid:', is_valid)
# 加密
ciphertext = rsa_encrypt(message, public_key)
print('ciphertext:', ciphertext)
# 解密
plaintext = rsa_decrypt(ciphertext, private_key)
print('plaintext:', plaintext)
```
这段代码使用了Python的`Crypto`库来实现RSA签名验签和加密解密功能。你可以根据自己的需求进行修改和调整。需要注意的是,这里的代码只是一个简单的示例,实际使用时需要保证数据的安全性和完整性。
阅读全文
相关推荐
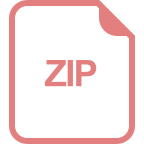
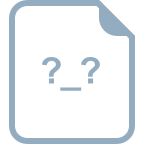
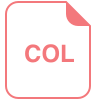
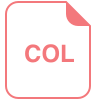
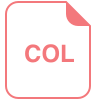
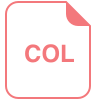
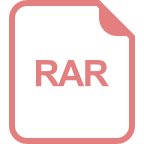
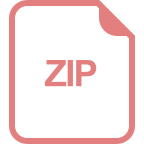
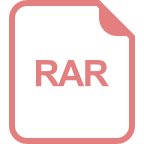
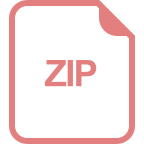
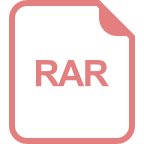
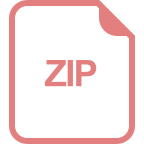
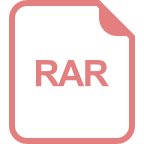