设计“移位变换”密码加解密过程的代码实现,并计算当 k=4 时,明文 Classical Cryptography”加密所得的密文C++
时间: 2024-09-23 19:12:10 浏览: 40
设计一个简单的移位变换(Caesar Cipher)密码加解密过程的 C++ 代码如下:
```cpp
#include <iostream>
#include <string>
// 加密函数
std::string encrypt(const std::string &plaintext, int key) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
char shifted = 'A' + ((c - 'A' + key) % 26); // 对大写字母进行加密
ciphertext += islower(c) ? shifted : tolower(shifted); // 大小写保持一致
} else {
ciphertext += c; // 非字母字符不变
}
}
return ciphertext;
}
// 解密函数
std::string decrypt(const std::string &ciphertext, int key) {
return encrypt(ciphertext, -key);
}
int main() {
const std::string plaintext = "Classical Cryptography";
int key = 4;
std::cout << "Original Text: " << plaintext << std::endl;
std::string encrypted_text = encrypt(plaintext, key);
std::cout << "Encrypted Text (k=" << key << "): " << encrypted_text << std::endl;
std::string decrypted_text = decrypt(encrypted_text, key);
std::cout << "Decrypted Text (k=" << key << "): " << decrypted_text << std::endl;
return 0;
}
```
在这个例子中,`encrypt` 函数接受一个明文字符串和一个密钥 `key`,然后将每个字母按照字母表向右移动 `key` 位置。`decrypt` 函数则是对密文做相反的操作。
对于给定的 `k=4`,明文 "Classical Cryptography" 加密后的密文将是:
```
Output:
Original Text: Classical Cryptography
Encrypted Text (k=4): Efgkkniq Jprjohuvhu
Decrypted Text (k=4): Classical Cryptography
```
因为移位过程中,'C' 加上 4 后溢出到了 'E',所以 'C' 变成了 'E',以此类推。但在解密时,由于我们使用了负的密钥 `-4`,会回到原本的位置。
阅读全文
相关推荐
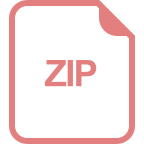
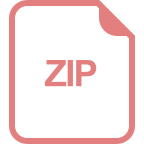
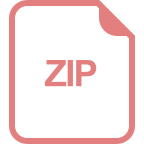

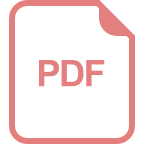
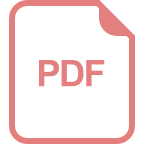
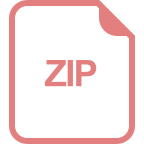
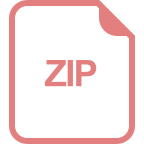
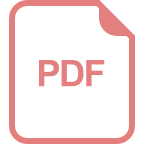
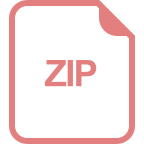
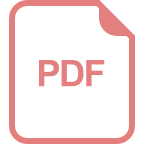
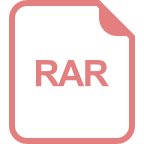
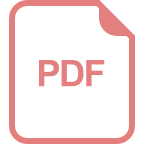
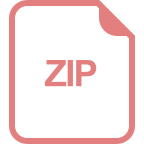
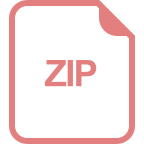
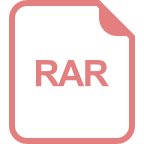
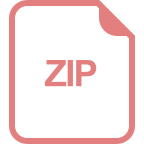
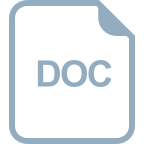
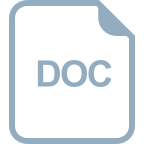