二叉树最深间隔最远结点C语言编写
时间: 2024-10-09 07:04:06 浏览: 60
二叉树中最深间隔最远的两个节点是指在树中找到两个节点,它们之间的路径长度最大,即路径上经过的边数最多。这个问题通常通过深度优先搜索(Depth First Search, DFS)解决,首先遍历整棵树,记录每个节点的最大深度和它的子节点的深度。
以下是使用C语言的基本思路:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 辅助函数,计算节点的深度
int max_depth(TreeNode* root) {
if (root == NULL) return 0;
int left_depth = max_depth(root->left);
int right_depth = max_depth(root->right);
return (left_depth > right_depth) ? left_depth + 1 : right_depth + 1;
}
// 找到最深间隔最远的两个节点
void find_nodes(TreeNode* root, int max_distance, int current_distance, TreeNode** node1, TreeNode** node2) {
if (root == NULL) return;
int this_distance = current_distance + 1;
if (*node1 == NULL || this_distance > (*node1)->depth) {
*node2 = *node1;
*node1 = root;
} else if (*node2 == NULL || this_distance > (*node2)->depth) {
*node2 = root;
}
find_nodes(root->left, max_distance, this_distance, node1, node2);
find_nodes(root->right, max_distance, this_distance, node1, node2);
}
// 主函数
int main() {
// 初始化二叉树...
TreeNode* root = ...; // 根据实际的二叉树构建
int max_distance = max_depth(root);
TreeNode* node1 = NULL, *node2 = NULL;
find_nodes(root, max_distance, 0, &node1, &node2);
printf("The two nodes with the maximum depth difference are:\n");
printf("Node 1 value: %d, Depth: %d\n", node1->val, node1->depth);
printf("Node 2 value: %d, Depth: %d\n", node2->val, node2->depth);
return 0;
}
```
注意,这个代码假设`TreeNode`结构包含了一个表示深度的字段`depth`。如果原始二叉树结构没有这样的信息,你需要在递归过程中计算并更新节点的深度。同时,这只是一个基本框架,实际应用中需要处理可能出现的边界情况,比如树为空的情况。
阅读全文
相关推荐





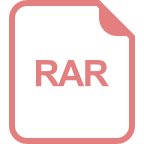





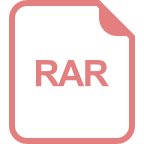
