用禁忌搜索算法,通过Python完成利用禁忌搜索算法解决旅行商问题,生成完整代码
时间: 2024-12-10 15:29:07 浏览: 13
禁忌搜索算法是一种启发式搜索算法,常用于求解复杂的优化问题,如旅行商问题(Traveling Salesman Problem, TSP)。旅行商问题是寻找一条路径,使得一位旅行商人访问所有城市一次,然后返回起点,总行程最短。
以下是使用Python实现禁忌搜索算法解决TSP的一个简单示例,我们将使用一个简单的版本,不涉及完整的详细编码,因为禁忌搜索有多种变体,例如LSA(Local Search Algorithm)版:
```python
import itertools
# 假设这是城市的列表
cities = [(0, 0), (5, 6), (3, 9), (7, 1), (8, 4)]
# 求解距离矩阵
def distance(city1, city2):
x1, y1 = city1
x2, y2 = city2
return ((x2 - x1) ** 2 + (y2 - y1) ** 2) ** 0.5
# 禁忌表初始为空
forbidden_set = set()
# 初始解
current_path = list(itertools.permutations(cities))
# 计算初始解的长度
best_solution = current_path[0]
best_distance = sum(distance(city1, city2) for city1, city2 in zip(current_path, current_path[1:] + current_path[:1]))
# 更新禁忌表
for path in forbidden_set:
if len(path) == len(best_solution) and distance(path[-1], path[0]) < best_distance:
break
while True:
# 获取当前路径的邻接解
neighbors = [path + [city] for path in current_path for city in cities if city not in path]
# 排除禁忌解
neighbors = [neighbor for neighbor in neighbors if neighbor not in forbidden_set]
# 计算邻居解的距离,并更新最好的解决方案
for neighbor in neighbors:
new_distance = sum(distance(city1, city2) for city1, city2 in zip(neighbor, neighbor[1:] + neighbor[:1]))
if new_distance < best_distance:
best_solution = neighbor
best_distance = new_distance
# 如果新解更好并且不在禁忌表里,加入禁忌表
if new_distance < best_distance and neighbor not in forbidden_set:
forbidden_set.add(neighbor)
else:
forbidden_set.remove(current_path)
# 如果找到最优解,提前结束循环
if len(forbidden_set) == 0:
break
current_path = best_solution
print(f"Best solution found: {best_solution}, Distance: {best_distance}")
#
阅读全文
相关推荐
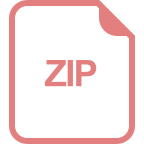
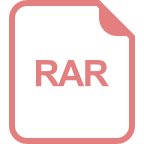
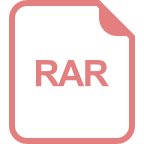

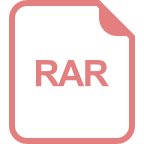
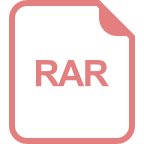



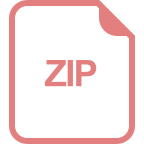
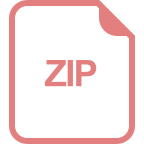
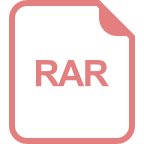
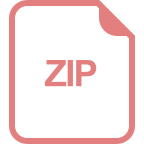
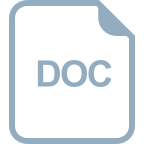
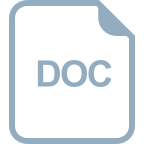

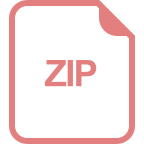
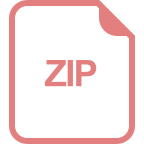