stream流根据某个属性去重
时间: 2024-03-01 13:46:34 浏览: 87
在Java中,可以使用Stream流根据某个属性去重。下面是一个示例代码:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Person> personList = new ArrayList<>();
personList.add(new Person("Alice", 25));
personList.add(new Person("Bob", 30));
personList.add(new Person("Alice", 25));
personList.add(new Person("Charlie", 35));
List<Person> distinctPersons = personList.stream()
.collect(Collectors.toMap(Person::getName, person -> person, (p1, p2) -> p1))
.values()
.stream()
.collect(Collectors.toList());
distinctPersons.forEach(person -> System.out.println(person.getName() + " - " + person.getAge()));
}
}
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
上述代码中,我们创建了一个Person类,包含name和age属性。我们使用Stream流对personList进行去重操作,根据name属性进行去重。具体步骤如下:
1. 使用`collect(Collectors.toMap(...))`将List转换为Map,其中key为name属性,value为对应的Person对象。
2. 使用`.values()`获取Map中的所有value,即去重后的Person对象集合。
3. 使用`collect(Collectors.toList())`将去重后的Person对象集合转换为List。
4. 最后,使用`forEach`遍历去重后的Person对象集合,并输出name和age属性。
运行上述代码,输出结果为:
```
Alice - 25
Bob - 30
Charlie - 35
```
阅读全文
相关推荐
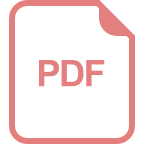
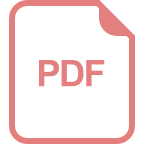
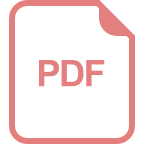















