创建3个重载的max方法,第一个返回两个整型参数中较大的一个;第二个返回两个双精度浮点数中较大的一个;第三个返回三个整数中最大的一个。
时间: 2024-10-29 22:14:25 浏览: 6
创建多个同名函数但参数列表不同的方法被称为方法重载(Overloading)。下面是一个简单的例子,展示了如何在Java中为`max`方法创建三个重载版本:
1. **整型版本**:
```java
public class MathUtils {
public int max(int a, int b) {
return a > b ? a : b;
}
}
```
2. **双精度浮点数版本**:
```java
public int max(double a, double b) { // 注意这里返回的是int类型的值,因为double到int会有舍入误差
int temp = (int)a;
if (temp > (int)b) return temp;
else return (int)b;
}
```
由于直接将双精度转换为整型可能会丢失精度,所以通常会先将double转换为int。
3. **三个整数版本**:
```java
public int max(int a, int b, int c) {
return Math.max(max(a, b), c);
}
```
这里利用了`Math.max()`函数,它接受任意数量的参数并返回其中的最大值。
相关问题
建立一个Java程序,学习创建和使用方法、掌握向方法 传递参数、学习掌握重载方法。程序要求:创建3个重载的max方法,第一个返回两个整型参数中较大的一个;第二个返回两个双精度浮点数中较大的一个;第三个返回三个整数中最大的一个。
在Java中,你可以通过定义同名但参数列表不同的函数来实现方法的重载(Overloading)。下面是一个简单的示例,展示了如何创建并使用三个名为`max`的方法,分别处理int、double和int数组的情况:
```java
public class MaxCalculator {
// 第一个重载的 max 方法,用于比较两个整数
public int max(int a, int b) {
return a > b ? a : b;
}
// 第二个重载的 max 方法,用于比较两个双精度浮点数
public double max(double a, double b) {
return a > b ? a : b;
}
// 第三个重载的 max 方法,用于比较三个整数
public int max(int a, int b, int c) {
int max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
return max;
}
// 测试这三个方法
public static void main(String[] args) {
MaxCalculator calculator = new MaxCalculator();
System.out.println("Max of 5 and 10 is: " + calculator.max(5, 10)); // 输出 10
System.out.println("Max of 2.5 and 3.7 is: " + calculator.max(2.5, 3.7)); // 输出 3.7
int[] numbers = {1, 4, 3, 6, 2};
System.out.println("Max of array elements is: " + calculator.max(numbers[0], numbers[1], numbers[2])); // 输出 6
}
}
```
在这个例子中,当你调用`max`方法时,Java会根据传入的实际参数类型自动选择相应版本的方法。
使用Java SDK建立一个Java程序,学习创建和使用方法、掌握向方法传递参数、学习掌握重载方法。程序要求:创建3个重载的max方法,第一个返回两个整型参数中较大的一个;第二个返回两个双精度浮点数中较大的一个;第三个返回三个整数中最大的一个。
在Java中,你可以通过定义不同的方法签名来实现方法的重载。下面是一个简单的例子,展示了如何创建和使用一个包含三个`max`方法的Java类:
```java
public class NumberMaxCalculator {
// 第一个 max 方法,接收两个整数并返回较大值
public int max(int a, int b) {
return Math.max(a, b);
}
// 第二个 max 方法,接收两个 double 并返回较大值
public double max(double a, double b) {
return Math.max(a, b);
}
// 第三个 max 方法,接收三个整数并返回最大值
public int max(int a, int b, int c) {
return Math.max(Math.max(a, b), c);
}
// 测试方法,演示如何使用这三个重载的 max 方法
public static void main(String[] args) {
NumberMaxCalculator calculator = new NumberMaxCalculator();
System.out.println("Max of 5 and 10: " + calculator.max(5, 10));
System.out.println("Max of 3.14 and 2.718: " + calculator.max(3.14, 2.718));
// 如果需要计算三个整数的最大值
int numbers[] = {1, 2, 3, 4, 5};
System.out.println("Max of three integers: " + calculator.max(numbers[0], numbers[1], numbers[2]));
}
}
```
在这个例子中,`NumberMaxCalculator`类有三个名为`max`的方法,它们各有不同的参数类型,因此可以被正确地根据传入的参数自动匹配调用。
阅读全文
相关推荐
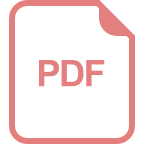
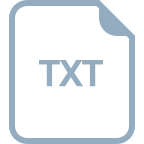
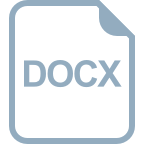

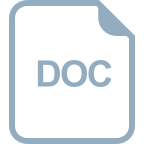
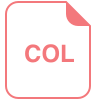
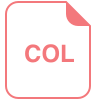
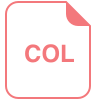
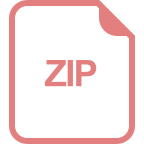
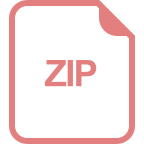
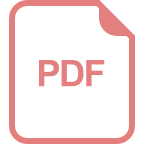
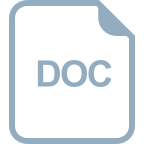
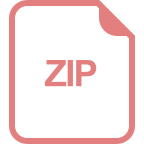