ElasticsearchClient和RestHighLevelClient区别
时间: 2023-11-08 15:04:20 浏览: 228
ElasticsearchClient和RestHighLevelClient是Java API中连接Elasticsearch的两个客户端,主要区别在于RestHighLevelClient相对于ElasticsearchClient提供了更加高级和全面的功能,例如支持异步操作、支持REST API的所有终端点、支持请求响应式编程等。
此外,RestHighLevelClient使用的是Java的高级REST客户端库,而ElasticsearchClient使用的是低级别的Java REST客户端库。
相关问题
JAVA ElasticsearchClient 8.0版本 elasticsearchClient 批量更新文档
在 Elasticsearch 8.0 版本中,可以使用 Java High Level REST Client 来进行批量更新文档操作。下面是一个示例代码:
```java
import org.apache.http.HttpHost;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentBuilder;
import org.elasticsearch.common.xcontent.XContentFactory;
import java.io.IOException;
public class ElasticsearchClientExample {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("localhost", 9200, "http")));
BulkRequest bulkRequest = new BulkRequest();
// 添加需要批量更新的文档
bulkRequest.add(new IndexRequest("your_index", "your_type", "document_id").source(createDocBuilder()));
BulkResponse bulkResponse = client.bulk(bulkRequest);
// 处理结果
if (bulkResponse.hasFailures()) {
// 批量更新失败
} else {
// 批量更新成功
}
client.close();
}
private static XContentBuilder createDocBuilder() throws IOException {
XContentBuilder builder = XContentFactory.jsonBuilder();
builder.startObject();
builder.field("field1", "value1");
builder.field("field2", "value2");
// 添加需要更新的字段和值
builder.field("field3", "value3");
builder.endObject();
return builder;
}
}
```
在上述示例中,使用 RestHighLevelClient 来与 Elasticsearch 进行通信。首先创建一个 BulkRequest 对象,然后使用 `bulkRequest.add()` 方法添加需要批量更新的文档,其中需要指定索引、类型和文档 ID,以及新的字段和值。最后执行 `client.bulk()` 方法进行批量更新,并根据返回结果判断更新是否成功。
请注意替换示例代码中的 "your_index"、"your_type" 和 "document_id" 为实际的索引、类型和文档 ID。另外,还需要根据需求添加更多的文档更新操作到 BulkRequest 对象中。
希望这个示例能够帮助到你!如果还有其他问题,请随时提问。
JAVA ElasticsearchClient 8.0版本 ElasticsearchClient update.action() 方法怎么用
在 JAVA ElasticsearchClient 8.0 版本中,ElasticsearchClient 类中的 update.action() 方法用于执行更新操作。该方法可以用于更新一个或多个文档的特定字段。
以下是使用 update.action() 方法的示例代码:
```java
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.action.update.UpdateResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
public class Main {
public static void main(String[] args) {
try (RestHighLevelClient client = new RestHighLevelClient(/* Elasticsearch 连接配置 */)) {
UpdateRequest request = new UpdateRequest("your_index", "your_document_id");
request.doc("{ \"field\": \"value\" }", XContentType.JSON);
UpdateResponse response = client.update(request, RequestOptions.DEFAULT);
System.out.println("Update result: " + response.getResult());
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述示例中,你需要替换以下参数:
- "your_index":你要更新的索引名称。
- "your_document_id":你要更新的文档 ID。
- "{ \"field\": \"value\" }":你要更新的字段及其新值。
你可以根据你的需求修改代码中的参数和字段值。执行该代码会发送一个更新请求到 Elasticsearch,然后你可以获取更新结果并进行相应的处理。
请注意,上述代码仅适用于 Elasticsearch 8.0 版本的 RestHighLevelClient。如果你使用其他版本或其他类型的客户端,请参考相应版本的文档进行调整。
阅读全文
相关推荐
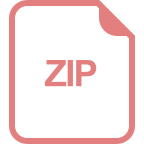
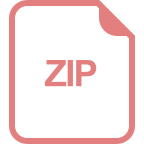













