用python语言输入年份year,输入天数n,判断该年的第n天是几月几日。(提示闰年2月29天:四年一闰,百年不闰,四百年再闰;平年2月28)
时间: 2024-10-11 11:08:33 浏览: 36
在Python中,你可以通过`calendar`模块来实现这个功能。首先需要确定给定年份是否为闰年,然后计算出从年初到指定日期的天数,最后根据这一天所在的位置确定月份和日期。以下是一个简单的函数实现:
```python
import calendar
def get_date(year, n):
# 判断闰年
is_leap = year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
# 确定每个月的天数
days_in_month = [calendar.monthrange(year, i)[1] for i in range(1, 13)]
# 计算第n天所在的月份和日期
day_index = n - sum(days_in_month[:month-1]) if is_leap else n - sum(days_in_month[:month])
month = 1 + day_index // days_in_month[day_index % months]
date = day_index % days_in_month[month - 1] + 1
return f"{month}月{date}日"
# 使用示例
year = int(input("请输入年份: "))
n = int(input("请输入天数: "))
print(get_date(year, n))
相关问题
python输入年份year,输入天数n,判断该年的第n天是几月几日。(提示闰年2月29天:四年一闰,百年不闰,四百年再闰;平年2月28)
在Python中,你可以通过编写一个函数来实现这个功能。首先,我们需要检查给定的年份是否是闰年,然后计算指定天数对应的月份和日期。下面是一个简单的示例:
```python
def day_of_year(year, n):
# 检查闰年规则
is_leap = (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0)
if is_leap:
days_in_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
total_days = sum(days_in_month[:int(n / 365)]) # 统计非闰年天数
if year > 1582: # 跳过格里高利历改革前的年份
for i in range(1583, year + 1): # 累加闰年的额外天数
if is_leap:
total_days += 1
# 计算剩余的天数和月份
remaining_days = n - total_days
month = remaining_days // days_in_month[1] + 1 # 因为2月份是最前面的
day = remaining_days % days_in_month[month - 1]
return f"{month}月{day}日"
# 测试
year = int(input("请输入年份: "))
n = int(input("请输入天数: "))
result = day_of_year(year, n)
print(f"该年的第{n}天是{result}")
```
编写一组python程序,输入年份year、月份month,判断该月的天数。闰年:能被4整除但不能被100整除或者能被400整除的年份是闰年。
可以使用以下代码实现:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
# 判断闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
leap_year = True
else:
leap_year = False
# 判断天数
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
else:
if leap_year:
days = 29
else:
days = 28
print("{}年{}月有{}天".format(year, month, days))
```
这段代码首先根据输入的年份判断是否为闰年,然后根据输入的月份判断该月的天数,并将结果输出。
阅读全文
相关推荐
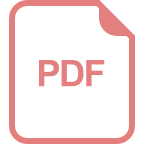
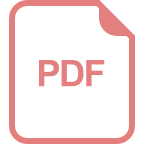
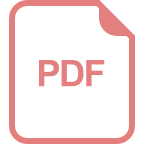














