Python VTK 交互
时间: 2023-11-19 11:49:42 浏览: 58
Python VTK交互是指使用Python语言与VTK(Visualization Toolkit)进行交互的过程。要在Python中使用VTK,首先需要安装VTK库。安装VTK库有多种方法,其中一种是使用Anaconda进行安装。可以通过conda安装命令来安装特定版本的VTK,例如:
```
conda install -n envA -c menpo vtk=7 python=3
```
另一种方法是直接下载VTK的whl文件并使用pip进行安装。你可以通过访问https://www.lfd.uci.edu/~gohlke/pythonlibs/#vtk来获取VTK的whl文件。选择适合你环境的版本进行下载,然后在下载目录中打开命令行,使用pip安装该文件,例如:
```
pip install VTK-7.1.1-cp36-cp36m-win_amd64.whl
```
安装完成后,就可以在Python中使用VTK库进行交互了。
相关问题
Python vtk交互
您好!对于Python和VTK的交互,您可以使用VTK提供的Python模块来实现。VTK(Visualization Toolkit)是一个强大的开源可视化库,可以用于创建和操作三维图形、图像处理和可视化数据。
要开始使用Python和VTK进行交互,首先需要安装VTK库。您可以使用pip命令来安装它:
```
pip install vtk
```
安装完成后,您可以在Python脚本中导入vtk模块:
```
import vtk
```
然后,您可以使用vtk模块中的各种类和函数来创建和操作VTK对象。例如,您可以创建一个vtkRenderWindow对象来显示可视化结果:
```
# 创建一个渲染窗口
render_window = vtk.vtkRenderWindow()
# 创建一个渲染器
renderer = vtk.vtkRenderer()
# 将渲染器添加到渲染窗口中
render_window.AddRenderer(renderer)
# 创建一个交互器并将其与渲染窗口关联
interactor = vtk.vtkRenderWindowInteractor()
interactor.SetRenderWindow(render_window)
# 渲染并启动交互器
render_window.Render()
interactor.Start()
```
这只是一个简单的例子,您还可以使用VTK进行更复杂的可视化操作,如加载和显示3D模型、创建体积渲染、进行图像处理等。您可以查阅VTK的官方文档和示例代码来了解更多详细信息。
希望这可以帮助到您!如果您有任何其他问题,请随时提问。
python vtk 拾取
您好!要在 Python 中使用 VTK(Visualization Toolkit)进行拾取(Picking),您可以按照以下步骤操作:
1. 导入所需的模块:
```python
import vtk
```
2. 创建 VTK 渲染窗口和交互器:
```python
renderer = vtk.vtkRenderer()
render_window = vtk.vtkRenderWindow()
render_window.AddRenderer(renderer)
interactor = vtk.vtkRenderWindowInteractor()
interactor.SetRenderWindow(render_window)
```
3. 创建一个拾取器对象并将其与渲染器关联:
```python
picker = vtk.vtkCellPicker()
picker.SetTolerance(0.005) # 设置拾取容差
picker.PickFromListOn() # 设置只拾取特定的 actor,若不需要则可忽略此行
renderer.SetPicker(picker)
```
4. 创建一个 VTK 模型(例如,一个球体):
```python
sphere_source = vtk.vtkSphereSource()
sphere_mapper = vtk.vtkPolyDataMapper()
sphere_mapper.SetInputConnection(sphere_source.GetOutputPort())
sphere_actor = vtk.vtkActor()
sphere_actor.SetMapper(sphere_mapper)
renderer.AddActor(sphere_actor)
```
5. 将所有组件添加到渲染窗口中并启动交互:
```python
render_window.Render()
interactor.Start()
```
6. 在交互器中注册拾取回调函数,并在回调函数中处理拾取事件:
```python
def on_pick(obj, event):
picker = obj.GetPicker()
picked_point = picker.GetPickPosition() # 获取拾取点的坐标
picked_actor = picker.GetActor() # 获取被拾取的 actor
if picked_actor:
print("Picked actor:", picked_actor)
interactor.AddObserver("LeftButtonPressEvent", on_pick) # 注册拾取回调函数
```
以上是一个简单的示例,您可以根据自己的需求进行调整和扩展。拾取器提供了其他有用的方法和属性,可以根据需要进行使用。希望对您有所帮助!如果您还有其他问题,请随时提问。
相关推荐
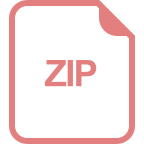
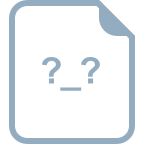
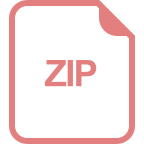












