按照注释中的要求,完善下列函数 #include<iostream> #define OK 1 #define ERROR 0 #define OVERFLOW -2 #define MVNum 100 //最大顶点数 using namespace std; typedef struct ArcNode {//边结点 int adjvex; //邻接点域:该边所指向的顶点的位置 int data; //数据域:存储和边相关的信息 struct ArcNode* nextarc; //链域:指向下一条边的指针 }ArcNode; typedef struct VNode {//顶点信息 int data; //顶点结点的数据域 ArcNode* firstarc; //链域:指向第一条依附该顶点的边的指针 }VNode,AdjList[MVNum]; //AdjList表示邻接表类型 typedef struct {//邻接表 AdjList vertices; int vexnum,arcnum; //图的当前顶点数和边数 }ALGragh; int CreateUDG(ALGragh &G,int vexnum,int arcnum) {//采用邻接表表示法,创建无向图G } void DeleteAdjList(VNode &List) {//删除指定顶点链表上的边结点 } int DeleteVex(ALGragh &G) {//删除G中顶点f及其相关的弧 } int PrintGraph(ALGragh G) {//输出图G }
时间: 2024-01-14 21:05:20 浏览: 38
在这个问题中,我们需要完善CreateUDG函数、DeleteAdjList函数、DeleteVex函数和PrintGraph函数。首先,我们需要根据邻接表类型的定义,重构CreateUDG函数:
```
int CreateUDG(ALGragh &G, int vexnum, int arcnum) {//采用邻接表表示法,创建无向图G
G.vexnum = vexnum;
G.arcnum = arcnum;
for (int i = 0; i < G.vexnum; i++) {
cout << "请输入第" << i + 1 << "个顶点的值:" << endl;
cin >> G.vertices[i].data;
G.vertices[i].firstarc = NULL;
}
for (int k = 0; k < G.arcnum; k++) {
int i, j;
cout << "请输入第" << k + 1 << "条边的顶点序号:" << endl;
cin >> i >> j;
ArcNode *p = new ArcNode;
p->adjvex = j - 1;
p->nextarc = G.vertices[i - 1].firstarc;
G.vertices[i - 1].firstarc = p;
ArcNode *q = new ArcNode;
q->adjvex = i - 1;
q->nextarc = G.vertices[j - 1].firstarc;
G.vertices[j - 1].firstarc = q;
}
return OK;
}
```
CreateUDG函数中,我们首先输入顶点的值,并将每个顶点的链表指针初始化为NULL。接着,我们输入每条边的顶点序号,新建两个边结点p和q,并分别将它们插入到对应的顶点链表中。
接下来,我们可以编写DeleteAdjList函数:
```
void DeleteAdjList(VNode &List) {//删除指定顶点链表上的边结点
ArcNode *p = List.firstarc, *q;
while (p != NULL) {
q = p;
p = p->nextarc;
delete q;
}
List.firstarc = NULL;
}
```
DeleteAdjList函数中,我们遍历指定顶点链表上的所有边结点,并逐个删除它们。最后,我们将该顶点链表的头指针设为NULL。
接下来,我们可以编写DeleteVex函数:
```
int DeleteVex(ALGragh &G) {//删除G中顶点f及其相关的弧
int f;
cout << "请输入要删除的顶点的值:" << endl;
cin >> f;
int i = -1;
//查找要删除的顶点的位置
for (int j = 0; j < G.vexnum; j++) {
if (G.vertices[j].data == f) {
i = j;
break;
}
}
if (i == -1) {//未找到要删除的顶点
return ERROR;
}
//删除以f为起点的边
DeleteAdjList(G.vertices[i]);
//删除以f为终点的边
for (int j = 0; j < G.vexnum; j++) {
ArcNode *p = G.vertices[j].firstarc, *q = NULL;
while (p != NULL) {
if (p->adjvex == i) {
if (q == NULL) {
G.vertices[j].firstarc = p->nextarc;
}
else {
q->nextarc = p->nextarc;
}
delete p;
p = q == NULL ? G.vertices[j].firstarc : q->nextarc;
}
else {
q = p;
p = p->nextarc;
}
}
}
//删除顶点f
for (int j = i; j < G.vexnum - 1; j++) {
G.vertices[j] = G.vertices[j + 1];
}
G.vexnum--;
//修改邻接表中顶点编号
for (int j = 0; j < G.vexnum; j++) {
ArcNode *p = G.vertices[j].firstarc;
while (p != NULL) {
if (p->adjvex > i) {
p->adjvex--;
}
p = p->nextarc;
}
}
return OK;
}
```
DeleteVex函数中,我们首先输入要删除的顶点的值,并查找该顶点在邻接表中的位置。接着,我们分别删除以该顶点为起点的边和以该顶点为终点的边,最后删除该顶点本身。在删除以该顶点为终点的边时,我们需要遍历邻接表中的每个顶点链表,找到以该顶点为终点的边,并删除它。在删除顶点f时,我们需要将后面的顶点向前移动一个位置,并修改邻接表中所有顶点的编号。
最后,我们可以编写PrintGraph函数:
```
int PrintGraph(ALGragh G) {//输出图G
for (int i = 0; i < G.vexnum; ++i) {
cout << G.vertices[i].data << ":";
ArcNode *p = G.vertices[i].firstarc;
while (p != NULL) {
cout << G.vertices[p->adjvex].data << "(" << p->data << ")" << "->";
p = p->nextarc;
}
cout << "NULL" << endl;
}
return OK;
}
```
PrintGraph函数中,我们遍历邻接表中的每个顶点链表,并输出它们的邻接点和权值。
完整代码如下:
相关推荐
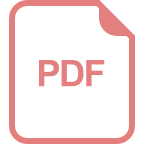
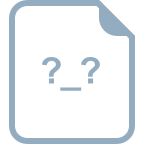
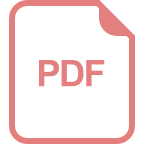
















