c++不用INIReader库读取配置文件内参数
时间: 2023-07-05 11:23:28 浏览: 112
除了使用第三方库之外,C++ 也提供了一些标准库来读取配置文件,比如 \<fstream\> 库和 \<sstream\> 库。下面是一种基于 \<fstream\> 库的读取配置文件的方法。
假设配置文件 config.ini 的内容如下:
```
[database]
host = localhost
port = 3306
username = root
password = 123456
```
可以使用以下代码读取配置文件:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <map>
int main() {
std::ifstream fin("config.ini");
std::map<std::string, std::map<std::string, std::string>> config;
std::string line;
std::string section;
while (std::getline(fin, line)) {
std::istringstream iss(line);
std::string key, value;
if (line[0] == '[' && line.back() == ']') {
section = line.substr(1, line.size() - 2);
} else if (std::getline(iss, key, '=') && std::getline(iss, value)) {
config[section][key] = value;
}
}
// 输出读取结果
for (const auto& section : config) {
std::cout << "[" << section.first << "]" << std::endl;
for (const auto& key_value : section.second) {
std::cout << key_value.first << " = " << key_value.second << std::endl;
}
std::cout << std::endl;
}
return 0;
}
```
这段代码使用了一个 std::map 对象来保存读取到的参数值,其中第一层的 key 是段落名称,第二层的 key-value 对是参数名和参数值。在读取文件时,首先使用 std::ifstream 打开文件,然后逐行读取文件内容。对于每一行,如果是段落名称,则保存该名称,如果是参数名和参数值,则保存到 map 对象中。
读取完文件之后,可以使用 map 对象来访问参数值。例如,访问 "database" 段中的 "username" 参数的值可以使用以下代码:
```c++
std::string username = config["database"]["username"];
```
需要注意的是,这种方法没有考虑配置文件中的注释和空行,如果需要支持注释和空行,需要在读取文件时进行额外的处理。
阅读全文
相关推荐


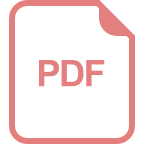
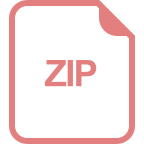
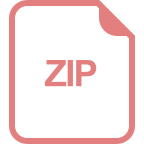
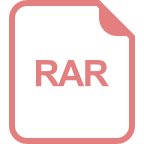
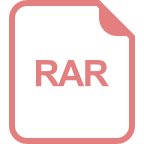
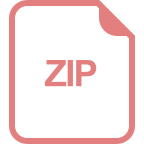
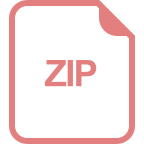
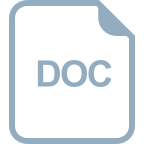
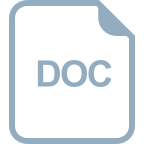



