c++中使用#include "INIReader.h"读取配置文件可以读取中文吗
时间: 2023-08-07 12:04:21 浏览: 83
INIReader 库默认是支持读取中文的,只需要保证配置文件的编码和 INIReader 库所使用的编码一致即可。INIReader 库默认使用 UTF-8 编码读取配置文件,因此需要确保配置文件也是以 UTF-8 编码保存的。如果你的配置文件使用了其他编码格式,需要先将其转换为 UTF-8 编码。
在读取配置文件时,可以使用 INIReader 的 GetString 方法来获取参数值:
```c++
std::string value = reader.GetString(section_name, key_name, default_value);
```
其中,section_name 是段落的名称,key_name 是参数的名称,default_value 是默认值。如果找不到对应的参数,则返回默认值。
如果你需要在代码中使用中文字符串,建议使用 UTF-8 编码保存源代码,并使用 std::string 类型来表示字符串。例如:
```c++
std::string str = "这是一个中文字符串";
```
可以直接将这个字符串保存到配置文件中,然后使用 INIReader 来读取。如果需要在程序中输出中文字符串,可以使用 std::cout 或者其他输出函数,但是需要确保控制台的编码和程序所使用的编码一致。
相关问题
C++编制一个读单词过程,以#include <stdio.h> #include <ctype.h> #include <stdlib.h> #include <string.h> #define NULL 0为开头
C++编写一个读取单词的过程可以以下代码:
```cpp
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
#define NULL 0
void readWord(char* word) {
int index = 0;
char ch;
// 跳过空白字符
while ((ch = getchar()) != EOF && isspace(ch));
// 读取单词字符
while (ch != EOF && !isspace(ch)) {
word[index++] = ch;
ch = getchar();
}
// 添加字符串结束符
word[index] = '\0';
}
int main() {
char word[100];
readWord(word);
printf("读取的单词是:%s\n", word);
return 0;
}
```
上述代码中,`readWord`函数用于读取一个单词,它会跳过输入中的空白字符,然后读取连续的非空白字符作为一个单词。`main`函数中调用了`readWord`函数,并打印出读取到的单词。
写一个INIReader.h
以下是一个简单的INIReader.h的实现,用于读取INI文件中的键值对:
```c++
#ifndef INIREADER_H
#define INIREADER_H
#include <string>
#include <map>
class INIReader
{
public:
INIReader(const std::string& filename);
std::string Get(const std::string& section, const std::string& name, const std::string& default_value = "") const;
private:
std::map<std::string, std::map<std::string, std::string>> data_;
};
#endif // INIREADER_H
```
实现细节:
1. 构造函数会读取INI文件中的所有键值对,并将它们存储在一个二维的map中,其中第一层map的key是section名,第二层map的key是name名,value是对应的值。这个数据结构可以方便地进行查询。
2. Get方法可以根据给定的section和name查找对应的值。如果找到了就返回对应的值,否则返回默认值。
下面是INIReader.cpp的实现:
```c++
#include "INIReader.h"
#include <fstream>
#include <algorithm>
INIReader::INIReader(const std::string& filename)
{
std::ifstream file(filename);
std::string line;
std::string current_section = "";
while (std::getline(file, line))
{
// 去掉行首和行尾的空格
line.erase(std::remove_if(line.begin(), line.end(), ::isspace), line.end());
if (line.empty() || line[0] == ';') // 空行或注释行
{
continue;
}
else if (line[0] == '[' && line[line.size() - 1] == ']') // section
{
current_section = line.substr(1, line.size() - 2);
}
else // name-value pair
{
auto pos = line.find('=');
if (pos != std::string::npos)
{
std::string name = line.substr(0, pos);
std::string value = line.substr(pos + 1);
data_[current_section][name] = value;
}
}
}
}
std::string INIReader::Get(const std::string& section, const std::string& name, const std::string& default_value) const
{
auto it1 = data_.find(section);
if (it1 != data_.end())
{
auto it2 = it1->second.find(name);
if (it2 != it1->second.end())
{
return it2->second;
}
}
return default_value;
}
```
实现细节:
1. 构造函数中使用了std::getline从文件中读取每一行,并使用std::isspace和std::remove_if去掉行首和行尾的空格。
2. 如果是空行或注释行,就直接跳过。
3. 如果是[section]行,就更新当前的section名。
4. 如果是name=value行,就将它存储在data_中对应的section和name下。
5. Get方法中首先查找给定的section是否存在,如果存在则在该section中查找给定的name是否存在,如果存在就返回对应的值,否则返回默认值。
阅读全文
相关推荐
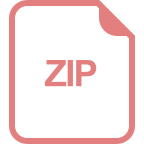
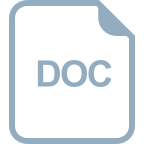

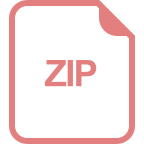
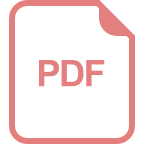
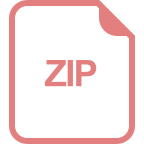
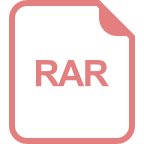
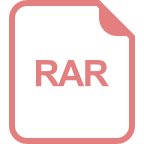
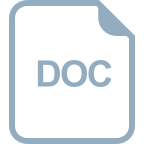





