qt create Linux类似qtconcurrent 函数
时间: 2024-03-21 10:40:28 浏览: 59
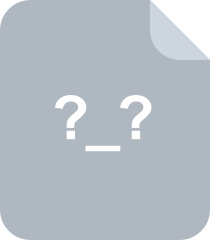
QT create for linux

在Linux下,可以使用POSIX线程库(pthread)来实现类似于QtConcurrent的函数。POSIX线程库是C语言的一套多线程支持库,可以在Linux、Unix、Mac OS X等多个平台上使用。使用pthread库需要包含头文件`<pthread.h>`,并使用相关的函数来创建、控制和销毁线程。
在使用pthread库时,需要注意线程安全和锁的问题,以避免多线程竞争导致的数据不一致和崩溃等问题。同时,需要注意线程的优先级和调度等问题,以充分利用多核CPU和提高程序性能。
下面是一个简单的例子,演示了如何使用pthread库来实现一个类似于QtConcurrent的函数:
```c++
#include <pthread.h>
#include <vector>
#include <algorithm>
template<typename T, typename Func>
void parallel_for_each(std::vector<T>& vec, Func func)
{
const size_t num_threads = 4; // 使用4个线程
const size_t chunk_size = vec.size() / num_threads;
std::vector<pthread_t> threads(num_threads);
std::vector<size_t> indices(num_threads + 1);
for (size_t i = 0; i <= num_threads; ++i)
{
indices[i] = i * chunk_size;
}
for (size_t i = 0; i < num_threads; ++i)
{
pthread_create(&threads[i], nullptr, [](void* arg) -> void*
{
auto* data = static_cast<std::pair<std::vector<T>*, Func>*>(arg);
const size_t start = data->first;
const size_t end = data->second;
auto& vec = *data->first;
auto& func = data->second;
for (size_t i = start; i < end; ++i)
{
func(vec[i]);
}
return nullptr;
}, &std::make_pair(&vec, func));
}
for (size_t i = 0; i < num_threads; ++i)
{
pthread_join(threads[i], nullptr);
}
}
int main()
{
std::vector<int> vec = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
parallel_for_each(vec, [](int& x) { x = x * x; });
std::for_each(vec.begin(), vec.end(), [](int x) { std::cout << x << " "; });
return 0;
}
```
该示例代码使用了4个线程来并行处理一个整数向量中的元素,将每个元素平方,并输出结果。其中,`parallel_for_each`函数是一个并行for_each函数,它将向量分成多个子向量,并在多个线程中并行地对每个子向量中的元素应用函数`func`。在每个线程中,使用`pthread_create`函数创建一个新线程,并使用lambda表达式来执行函数`func`。在主线程中,使用`pthread_join`函数等待所有线程结束。
阅读全文
相关推荐
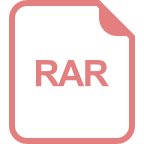
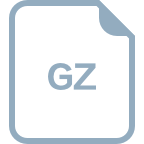
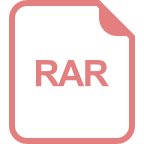
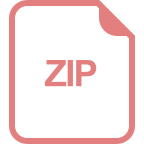
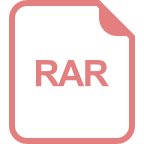
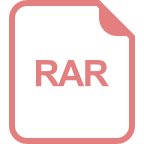
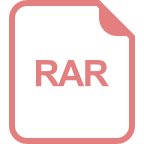
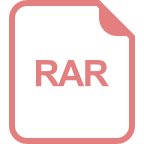
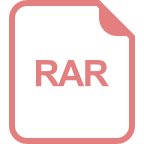
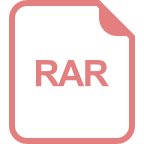
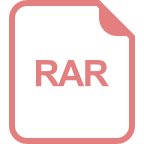
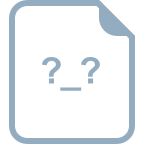
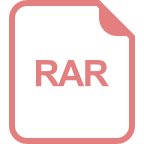