在Qt5环境中,如何解析从UDP接收的ArrayBuffer数据,特别是其中包含JSON格式的地理位置信息?能否提供一个详尽和完整的代码示例以说明这个过程?在主函数中实现
时间: 2024-10-23 20:10:36 浏览: 10
在Qt5中,解析从UDP收到的ArrayBuffer数据并提取JSON地理位置信息通常涉及以下几个步骤:
1. 首先,我们需要创建一个UDP socket,并监听特定端口。
2. 接收数据后,将其转换为QByteArray。
3. 使用QJsonDocument来解析JSON数据。
4. 提取地理位置信息,如经纬度。
以下是完整的代码示例:
```cpp
#include <QObject>
#include <QTcpSocket>
#include <QUdpSocket>
#include <QJsonDocument>
#include <QJsonObject>
#include <QDebug>
class UdpReceiver : public QObject {
Q_OBJECT
public slots:
void startListening(int port) {
QUdpSocket *udpSocket = new QUdpSocket(this);
if (!udpSocket->bind(port)) {
qDebug() << "Failed to bind UDP socket to port" << port;
return;
}
connect(udpSocket, &QUdpSocket::readyRead, this, &UdpReceiver::handleData);
qDebug() << "Listening on port" << port;
}
private slots:
void handleData() {
QByteArray buffer = udpSocket->readAll();
QBuffer bufferStream(buffer);
bufferStream.open(QIODevice::ReadOnly);
if (buffer.isEmpty()) {
qDebug() << "Received empty data";
return;
}
QJsonDocument jsonDoc = QJsonDocument::fromJson(buffer);
if (!jsonDoc.isObject()) {
qDebug() << "Invalid JSON format";
return;
}
const QJsonObject jsonObject = jsonDoc.object();
QJsonObject locationObj = jsonObject.value("location").toObject();
double latitude = locationObj.value("latitude", -1).toDouble();
double longitude = locationObj.value("longitude", -1).toDouble();
if (latitude != -1 && longitude != -1) {
qDebug() << "Received location: Latitude: " << latitude << ", Longitude: " << longitude;
} else {
qDebug() << "Location not found in the JSON object.";
}
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
UdpReceiver receiver;
receiver.startListening(8000); // Replace with your desired port
return app.exec();
}
```
在这个例子中,`startListening`方法用于启动UDP监听,`handleData`槽函数则负责接收数据并在接收到数据后尝试解析JSON。如果找到名为"location"的对象,它会提取出经纬度值。注意,实际应用中可能需要更复杂的错误检查和数据验证。
阅读全文
相关推荐
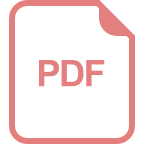
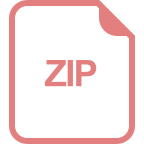
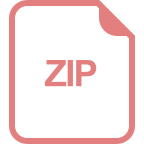














