图书管理系统设计与实现单链表
时间: 2023-08-14 15:03:27 浏览: 119
好的,我可以和你讨论关于图书管理系统设计与实现单链表的问题。
首先,单链表是一种常见的数据结构,用于存储一系列的数据元素。在图书管理系统中,我们可以使用单链表来存储图书信息。
单链表由多个节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。在图书管理系统中,每个节点可以存储一本书的信息,例如书名、作者、出版社、ISBN号等等。
我们可以使用一个头指针来指向链表的第一个节点。通过遍历链表,我们可以实现对图书信息的增、删、改、查等操作。
下面是一个简单的示例代码,用于实现单链表存储图书信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Book {
char title[50];
char author[50];
char publisher[50];
char isbn[20];
struct Book *next;
};
struct Book *head = NULL;
void add_book() {
struct Book *new_book = malloc(sizeof(struct Book));
printf("Please enter the book title: ");
scanf("%s", new_book->title);
printf("Please enter the book author: ");
scanf("%s", new_book->author);
printf("Please enter the book publisher: ");
scanf("%s", new_book->publisher);
printf("Please enter the book ISBN: ");
scanf("%s", new_book->isbn);
new_book->next = NULL;
if (head == NULL) {
head = new_book;
} else {
struct Book *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_book;
}
printf("Book added successfully!\n");
}
void list_books() {
if (head == NULL) {
printf("No books in the library!\n");
return;
}
printf("%-50s %-50s %-50s %-20s\n", "Title", "Author", "Publisher", "ISBN");
struct Book *current = head;
while (current != NULL) {
printf("%-50s %-50s %-50s %-20s\n", current->title, current->author, current->publisher, current->isbn);
current = current->next;
}
}
void search_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to search: ");
scanf("%s", isbn);
struct Book *current = head;
while (current != NULL) {
if (strcmp(current->isbn, isbn) == 0) {
printf("%-50s %-50s %-50s %-20s\n", "Title", "Author", "Publisher", "ISBN");
printf("%-50s %-50s %-50s %-20s\n", current->title, current->author, current->publisher, current->isbn);
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
void delete_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to delete: ");
scanf("%s", isbn);
if (head == NULL) {
printf("No books in the library!\n");
return;
}
if (strcmp(head->isbn, isbn) == 0) {
struct Book *temp = head;
head = head->next;
free(temp);
printf("Book deleted successfully!\n");
return;
}
struct Book *current = head;
while (current->next != NULL) {
if (strcmp(current->next->isbn, isbn) == 0) {
struct Book *temp = current->next;
current->next = current->next->next;
free(temp);
printf("Book deleted successfully!\n");
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
void update_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to update: ");
scanf("%s", isbn);
struct Book *current = head;
while (current != NULL) {
if (strcmp(current->isbn, isbn) == 0) {
printf("Please enter the new book title: ");
scanf("%s", current->title);
printf("Please enter the new book author: ");
scanf("%s", current->author);
printf("Please enter the new book publisher: ");
scanf("%s", current->publisher);
printf("Please enter the new book ISBN: ");
scanf("%s", current->isbn);
printf("Book updated successfully!\n");
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
int main() {
int option;
do {
printf("Please select an option:\n");
printf("1. Add a book to the library\n");
printf("2. List all books in the library\n");
printf("3. Search for a book by ISBN\n");
printf("4. Delete a book from the library\n");
printf("5. Update a book in the library\n");
printf("6. Exit\n");
printf("Your selection: ");
scanf("%d", &option);
switch (option) {
case 1:
add_book();
break;
case 2:
list_books();
break;
case 3:
search_book();
break;
case 4:
delete_book();
break;
case 5:
update_book();
break;
case 6:
printf("Goodbye!\n");
break;
default:
printf("Invalid option!\n");
break;
}
} while (option != 6);
return 0;
}
```
在这个示例代码中,我们定义了一个Book结构体,包含了书的各个信息和指向下一个节点的指针。我们还定义了一个头指针head,用于指向链表的第一个节点。
我们通过add_book()函数实现了向链表中添加图书的功能,通过list_books()函数实现了列出所有图书的功能,通过search_book()函数实现了通过ISBN号搜索图书的功能,通过delete_book()函数实现了删除指定图书的功能,通过update_book()函数实现了更新指定图书信息的功能。
在main()函数中,我们通过一个循环来实现了用户与程序的交互,让用户能够选择相应的操作。
相关推荐
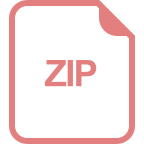
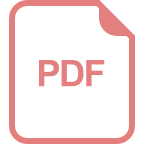
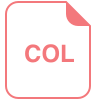
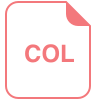
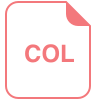
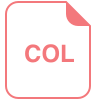
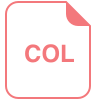







