python 文字转语音
时间: 2023-07-03 14:13:16 浏览: 113
在Python中,可以通过调用第三方语音合成API实现文字转语音功能,例如Google Text-to-Speech、Microsoft Azure Speech、Amazon Polly等等。
以下是使用Google Text-to-Speech进行文字转语音的示例代码:
```python
# 引入Google Text-to-Speech的Python库
from gtts import gTTS
import os
# 定义待转化的文字内容
text = "Hello, I am an AI assistant."
# 调用Google Text-to-Speech API进行语音合成
tts = gTTS(text)
tts.save("audio.mp3")
# 播放生成的语音文件
os.system("mpg321 audio.mp3")
```
该示例代码中,我们首先引入了Google Text-to-Speech的Python库,然后定义了待转化的文字内容,并调用`tts = gTTS(text)`将文字转化为语音。接着,我们将生成的语音文件保存到本地,并调用`os.system("mpg321 audio.mp3")`进行语音播放。这里我们使用了Linux系统上常用的播放器mpg321,如果您使用的是其他操作系统或者播放器,则需要相应地进行更改。
相关问题
python文字转语音停顿
根据提供的引用内容,可以看出您想了解的是Python中文字转语音停顿的相关知识。在Python中,可以使用Text-to-Speech (TTS)库来实现文字转语音的功能。其中,常用的TTS库有pyttsx3、gTTS等。这些库都可以实现文字转语音的功能,并且可以设置语速、音量等参数。如果您想要实现文字转语音停顿的功能,可以使用pyttsx3库中的pause()方法。该方法可以在语音输出时暂停一段时间,从而实现停顿的效果。下面是一个简单的示例代码:
```
import pyttsx3
# 创建TTS引擎
engine = pyttsx3.init()
# 设置语速
engine.setProperty('rate', 150)
# 设置音量
engine.setProperty('volume', 0.7)
# 输出文字
engine.say('Hello, world!')
# 停顿1秒
engine.pause(1)
# 输出文字
engine.say('How are you today?')
# 停顿2秒
engine.pause(2)
# 运行引擎
engine.runAndWait()
```
上述代码中,我们使用了pyttsx3库创建了一个TTS引擎,并设置了语速和音量。然后,我们使用engine.say()方法输出了两段文字,并使用engine.pause()方法在输出文字时暂停了一段时间,从而实现了停顿的效果。
Python 文字转语音免费
要在 Python 中进行文字转语音,你可以使用 Google Text-to-Speech API。Google Text-to-Speech API 允许你通过提供一个文本字符串来生成一个音频文件,该音频文件可以是 WAV 或 MP3 格式。
以下是一个简单的 Python 代码示例,演示如何使用 Google Text-to-Speech API 将文本转换为语音:
```python
from google.cloud import texttospeech
# Replace with your Google Cloud project ID
project_id = 'your-project-id'
# Create the Text-to-Speech client
client = texttospeech.TextToSpeechClient()
# Set the text input to be synthesized
synthesis_input = texttospeech.SynthesisInput(text='Hello, world!')
# Build the voice request
voice = texttospeech.VoiceSelectionParams(
language_code='en-US',
name='en-US-Wavenet-D'
)
# Select the type of audio file you want returned
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
# Perform the text-to-speech request
response = client.synthesize_speech(
input=synthesis_input,
voice=voice,
audio_config=audio_config
)
# Save the audio file
with open('output.mp3', 'wb') as out:
out.write(response.audio_content)
print('Audio content written to file "output.mp3"')
```
请注意,使用 Google Text-to-Speech API 需要你拥有一个 Google Cloud Platform 帐号,并且需要设置适当的身份验证和授权。Google 提供了一定的免费配额,但在超出免费配额后,你可能需要支付一定的费用。
阅读全文
相关推荐
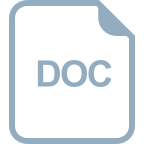
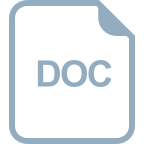
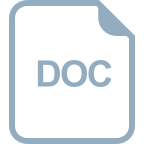

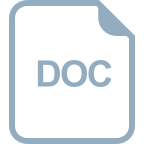
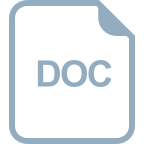
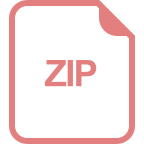
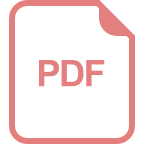
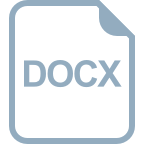
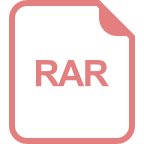
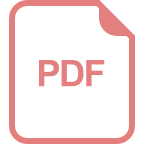
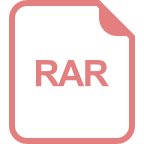
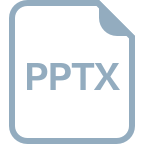