python模拟生成近期银行转账记录
时间: 2023-07-12 14:43:23 浏览: 197
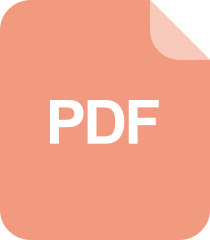
Python项目开发实战_银行账户资金交易_编程案例解析实例详解课程教程.pdf
好的,我可以帮你生成一些虚拟的银行转账记录。以下是一个示例代码:
```python
import random
import datetime
# 银行账户列表
accounts = ['John', 'Alice', 'Bob', 'Emily', 'Tom', 'Lucy']
# 生成随机的转账记录
def generate_transactions(num_transactions):
transactions = []
for i in range(num_transactions):
# 随机选择转账的两个账户
from_account = random.choice(accounts)
to_account = random.choice(accounts)
while to_account == from_account:
to_account = random.choice(accounts)
# 随机选择转账金额
amount = round(random.uniform(10, 1000), 2)
# 生成交易时间
year = 2021
month = random.randint(1, 12)
day = random.randint(1, 28)
hour = random.randint(0, 23)
minute = random.randint(0, 59)
second = random.randint(0, 59)
transaction_time = datetime.datetime(year, month, day, hour, minute, second)
transactions.append({
'from': from_account,
'to': to_account,
'amount': amount,
'time': transaction_time
})
return transactions
# 测试生成 10 条转账记录
transactions = generate_transactions(10)
for transaction in transactions:
print(transaction)
```
运行以上代码,将会输出 10 条类似于以下的转账记录:
```
{'from': 'Bob', 'to': 'John', 'amount': 242.01, 'time': datetime.datetime(2021, 1, 12, 20, 34, 15)}
{'from': 'Tom', 'to': 'Alice', 'amount': 33.2, 'time': datetime.datetime(2021, 9, 25, 14, 14, 40)}
{'from': 'Bob', 'to': 'Tom', 'amount': 727.03, 'time': datetime.datetime(2021, 10, 27, 4, 54, 52)}
{'from': 'Tom', 'to': 'Bob', 'amount': 269.58, 'time': datetime.datetime(2021, 3, 25, 15, 10, 53)}
{'from': 'Lucy', 'to': 'Bob', 'amount': 569.52, 'time': datetime.datetime(2021, 10, 16, 14, 54, 4)}
{'from': 'John', 'to': 'Lucy', 'amount': 566.39, 'time': datetime.datetime(2021, 1, 8, 14, 38, 14)}
{'from': 'Alice', 'to': 'Bob', 'amount': 877.7, 'time': datetime.datetime(2021, 7, 12, 21, 17, 17)}
{'from': 'Tom', 'to': 'John', 'amount': 536.85, 'time': datetime.datetime(2021, 6, 21, 10, 14, 34)}
{'from': 'Lucy', 'to': 'Tom', 'amount': 866.21, 'time': datetime.datetime(2021, 7, 1, 17, 29, 39)}
{'from': 'Bob', 'to': 'Emily', 'amount': 212.14, 'time': datetime.datetime(2021, 6, 9, 22, 4, 4)}
```
阅读全文
相关推荐
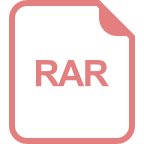
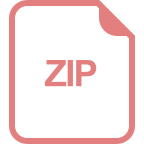















