std:map count 判断是否包含
时间: 2023-11-09 19:59:58 浏览: 19
可以使用 std::map 的 count 方法来判断一个 key 是否存在于 map 中。count 方法返回的是一个整数值,表示 key 出现的次数,如果 key 存在,则返回 1,否则返回 0。因此,可以根据 count 方法的返回值来判断 key 是否存在于 map 中。例如:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap{{"apple", 1}, {"banana", 2}, {"orange", 3}};
// 判断 key 是否存在
if (myMap.count("apple")) {
std::cout << "myMap contains key 'apple'" << std::endl;
} else {
std::cout << "myMap does not contain key 'apple'" << std::endl;
}
return 0;
}
```
输出:
```
myMap contains key 'apple'
```
相关问题
std::map count
`std::map` 是 C++ 标准库中的关联容器之一,它提供了一种键-值对的映射关系。`std::map` 中的每个元素都由一个键和一个值组成,键是唯一的,用于快速查找和访问对应的值。
要计算 `std::map` 中特定键的数量,可以使用 `count` 成员函数。`count` 函数接受一个键作为参数,并返回该键在 `std::map` 中的出现次数。如果该键存在,则返回 1;如果不存在,则返回 0。
以下是一个使用 `count` 函数的示例:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, int> myMap;
myMap[1] = 10;
myMap[2] = 20;
myMap[3] = 30;
myMap[4] = 20;
int key = 2;
int count = myMap.count(key);
std::cout << "Number of occurrences of key " << key << ": " << count << std::endl;
return 0;
}
```
在上述示例中,我们创建了一个 `std::map`,并插入了几个键值对。然后,我们使用 `count` 函数计算键为 2 的元素出现的次数,并将结果打印输出。
希望能解答你的问题!如果还有其他疑问,请随时提问。
std::map的count方法的功能
`std::map` 是 C++ 标准库中的关联容器,它提供了一种键值对的存储方式,并按照键的顺序进行排序。`std::map` 中的 `count` 方法用于统计给定键在 `map` 中出现的次数。
`count` 方法的功能是返回给定键在 `map` 中的出现次数。如果给定键存在于 `map` 中,则返回 1;如果给定键不存在,则返回 0。
下面是 `std::map` 的 `count` 方法的用法示例:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, int> myMap;
myMap[1] = 10;
myMap[2] = 20;
myMap[3] = 30;
int key = 2;
int count = myMap.count(key);
std::cout << "The count of key " << key << " is: " << count << std::endl;
return 0;
}
```
输出结果为:
```
The count of key 2 is: 1
```
在上述示例中,我们创建了一个 `std::map` 对象 `myMap`,并插入了三个键值对。然后,我们使用 `count` 方法统计键为 2 的出现次数,得到结果为 1。
请注意,`std::map` 是按照键进行排序的,因此键的重复次数只会是 0 或 1。如果您需要统计某个键在 `std::map` 中出现的次数大于 1,可能需要考虑使用 `std::multimap` 或其他数据结构。
相关推荐
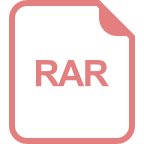
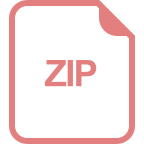
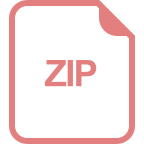













