编程设计一个简单的程序计算: n!=1*2*3*...*n
时间: 2024-10-17 11:01:07 浏览: 1
要编写一个简单的C++程序来计算阶乘(n!),你可以使用循环结构来相乘从1到n的所有整数。这里有一个基础的递归版本以及非递归版本的示例:
**递归版本:**
```cpp
#include <iostream>
// 定义阶乘函数,使用递归
unsigned long long factorial(int n) {
if (n == 0 || n == 1) { // 阶乘的基本情况
return 1;
} else {
return n * factorial(n - 1); // 递归调用自身
}
}
int main() {
int n;
std::cout << "Enter a non-negative integer: ";
std::cin >> n;
if (n < 0) {
std::cout << "Error: Factorial is not defined for negative numbers." << std::endl;
} else {
unsigned long long result = factorial(n);
std::cout << "Factorial of " << n << " is: " << result << std::endl;
}
return 0;
}
```
**非递归版本(使用循环):**
```cpp
#include <iostream>
// 定义阶乘函数,使用循环
unsigned long long factorial(int n) {
unsigned long long result = 1;
for (int i = 1; i <= n; ++i) {
result *= i;
}
return result;
}
int main() {
int n;
std::cout << "Enter a non-negative integer: ";
std::cin >> n;
if (n < 0) {
std::cout << "Error: Factorial is not defined for negative numbers." << std::endl;
} else {
unsigned long long result = factorial(n);
std::cout << "Factorial of " << n << " is: " << result << std::endl;
}
return 0;
}
```
这两个版本都可以计算给定数字n的阶乘,但请注意,对于较大的n值,递归可能会因为栈溢出而受限。
阅读全文
相关推荐
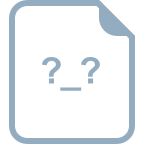
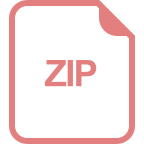
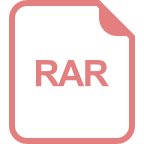
















