Stm32103c8与lcd1602的程序代码
时间: 2023-10-09 10:15:02 浏览: 36
以下是一个基于STM32F103C8和LCD1602的程序代码示例:
```c
#include "stm32f10x.h"
#include "delay.h"
// 定义LCD控制引脚
#define LCD_RS PBout(12)
#define LCD_RW PBout(11)
#define LCD_EN PBout(10)
#define LCD_D4 PBout(5)
#define LCD_D5 PBout(4)
#define LCD_D6 PBout(3)
#define LCD_D7 PBout(2)
// 初始化LCD
void LCD_Init(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); // 使能GPIOB时钟
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_10 | GPIO_Pin_11 | GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
LCD_EN = 0;
LCD_RS = 0;
LCD_RW = 0;
delay_ms(15);
LCD_WriteCommand(0x30);
delay_ms(5);
LCD_WriteCommand(0x30);
delay_ms(1);
LCD_WriteCommand(0x30);
delay_ms(1);
LCD_WriteCommand(0x20);
delay_ms(1);
LCD_WriteCommand(0x28);
delay_ms(1);
LCD_WriteCommand(0x08);
delay_ms(1);
LCD_WriteCommand(0x01);
delay_ms(1);
LCD_WriteCommand(0x06);
delay_ms(1);
LCD_WriteCommand(0x0C);
}
// 写命令
void LCD_WriteCommand(uint8_t command)
{
LCD_RS = 0;
LCD_RW = 0;
LCD_EN = 1;
LCD_D7 = command & 0x80;
LCD_D6 = command & 0x40;
LCD_D5 = command & 0x20;
LCD_D4 = command & 0x10;
LCD_EN = 0;
delay_us(1);
LCD_EN = 1;
LCD_D7 = command & 0x08;
LCD_D6 = command & 0x04;
LCD_D5 = command & 0x02;
LCD_D4 = command & 0x01;
LCD_EN = 0;
delay_us(1);
}
// 写数据
void LCD_WriteData(uint8_t data)
{
LCD_RS = 1;
LCD_RW = 0;
LCD_EN = 1;
LCD_D7 = data & 0x80;
LCD_D6 = data & 0x40;
LCD_D5 = data & 0x20;
LCD_D4 = data & 0x10;
LCD_EN = 0;
delay_us(1);
LCD_EN = 1;
LCD_D7 = data & 0x08;
LCD_D6 = data & 0x04;
LCD_D5 = data & 0x02;
LCD_D4 = data & 0x01;
LCD_EN = 0;
delay_us(1);
}
// 显示字符串
void LCD_ShowString(uint8_t x, uint8_t y, uint8_t *str)
{
uint8_t i = 0;
if (y == 0) {
LCD_WriteCommand(0x80 + x);
} else {
LCD_WriteCommand(0xC0 + x);
}
while (str[i] != '\0') {
LCD_WriteData(str[i]);
i++;
}
}
int main(void)
{
LCD_Init();
while (1) {
LCD_ShowString(0, 0, "Hello, World!");
delay_ms(1000);
LCD_WriteCommand(0x01);
delay_ms(500);
}
}
```
这是一个简单的LCD驱动程序,使用了STM32F103C8芯片,GPIOB口控制LCD1602。程序中定义了一些宏来控制LCD的各个引脚,包括RS、RW、EN、D4、D5、D6、D7。在初始化LCD时,先将控制引脚设为输出模式,并执行一些初始化命令来设置LCD的工作模式。然后可以调用`LCD_WriteCommand`和`LCD_WriteData`函数来写入命令和数据。最后,可以使用`LCD_ShowString`函数来显示字符串。程序中还包括了一些延时函数,保证信号稳定。
相关推荐
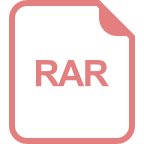
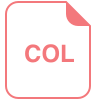
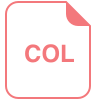
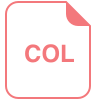
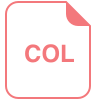
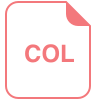










