写一段降采样的c语言代码
时间: 2023-12-15 12:55:48 浏览: 61
以下是一个简单的降采样函数,将输入的数组下采样一定倍数,返回下采样后的数组:
```
int* downsample(int* input, int input_len, int factor) {
int output_len = input_len / factor;
int* output = malloc(output_len * sizeof(int));
int i, j;
for (i = 0; i < output_len; i++) {
int sum = 0;
for (j = 0; j < factor; j++) {
sum += input[i * factor + j];
}
output[i] = sum / factor;
}
return output;
}
```
函数接收三个参数:输入数组、输入数组长度和下采样因子。它首先计算输出数组的长度,然后为它分配内存。接下来,它使用两个循环来计算每个输出值:外部循环迭代输出数组,内部循环迭代输入数组的一系列元素,将它们相加并将和除以下采样因子。最后返回输出数组。
这个函数假定输入数组长度是下采样因子的倍数,因为它将输入数组划分为一系列大小为下采样因子的子数组。如果输入数组长度不能被下采样因子整除,那么它将忽略最后几个元素。如果需要,可以在函数中添加额外的逻辑来处理这种情况。
相关问题
写一段2倍降采样的c语言代码
以下是一段2倍降采样的C语言代码:
```c
#include <stdio.h>
void downsample(int *in, int *out, int len) {
int i;
for (i = 0; i < len; i += 2) {
out[i / 2] = (in[i] + in[i + 1]) / 2;
}
}
int main() {
int in[] = {1, 2, 3, 4, 5, 6, 7, 8};
int out[4];
downsample(in, out, 8);
int i;
for (i = 0; i < 4; i++) {
printf("%d ", out[i]);
}
printf("\n");
return 0;
}
```
这段代码接受一个输入数组和一个输出数组,将输入数组的长度视为偶数,将相邻两个元素的平均值存储到输出数组中。例如,如果输入数组是{1, 2, 3, 4, 5, 6, 7, 8},那么输出数组将是{1.5, 3.5, 5.5, 7.5}。
用c语言,实现一个对音频降采样的方法
好的,以下是使用C语言实现的对音频降采样的方法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
void downsample(int16_t* in_buf, int16_t* out_buf, int in_size, int out_size) {
int i, j;
int ratio = in_size / out_size;
for (i = 0, j = 0; j < out_size; i += ratio, j++) {
out_buf[j] = in_buf[i];
}
}
int main() {
FILE *in_file = fopen("input.pcm", "rb");
FILE *out_file = fopen("output.pcm", "wb");
if (!in_file || !out_file) {
printf("Failed to open file.\n");
return -1;
}
int16_t in_buf[1024];
int16_t out_buf[512];
while (fread(in_buf, sizeof(int16_t), 1024, in_file) == 1024) {
downsample(in_buf, out_buf, 1024, 512);
fwrite(out_buf, sizeof(int16_t), 512, out_file);
}
fclose(in_file);
fclose(out_file);
return 0;
}
```
这段代码会从文件中读取音频采样数据,每次读取1024个采样点,并将其传递给`downsample`函数进行降采样。`downsample`函数会将输入缓冲区中的采样点按照指定的降采样比例进行筛选,并将筛选后的采样点存储到输出缓冲区中。最后,它会将输出缓冲区中的降采样数据写入到文件中。在本例中,输入采样率为44100 Hz,输出采样率为22050 Hz。
需要注意的是,本例中的代码仅适用于采样位深度为16位的音频数据。如果您的音频数据采样位深度不同,请修改代码中涉及到`int16_t`类型的部分。
阅读全文
相关推荐
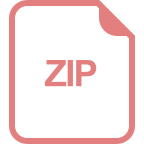
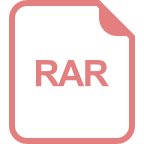
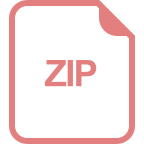
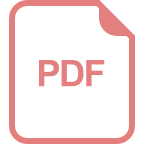
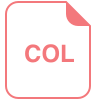
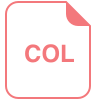
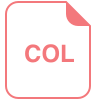
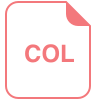
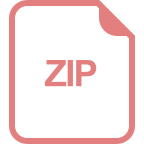
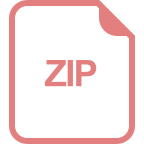