dgllife.model.gnn与torch_geometric.nn.GraphConv输入的参数以及区别
时间: 2024-06-02 07:11:26 浏览: 244
DGL和PyTorch Geometric都是用于图神经网络(GNN)的库,其中DGL提供了dgllife.model.gnn模块,而PyTorch Geometric提供了torch_geometric.nn.GraphConv模块。
在这两个模块中,输入参数和输出参数的定义非常相似。它们都需要输入图结构数据(通常由节点特征矩阵和边列表组成),然后产生新的节点特征矩阵作为输出。
然而,这两个模块之间有一些区别。其中一个主要区别是它们使用的库不同。DGL使用了自己的图形库,而PyTorch Geometric使用了PyTorch的Tensor库。这意味着在使用这些模块时,你需要确保你的输入数据类型与使用的库匹配。
另一个区别是这些模块中的GraphConv实现方式不同。DGL中的GraphConv实现与PyTorch Geometric中的GraphConv实现略有不同。但是,它们都是基于图卷积网络(GCN)的实现,用于在图结构上执行节点特征传递。
总的来说,这两个模块都是用于GNN的基本模块,可以用于构建各种类型的GNN模型。由于它们的实现略有不同,因此在使用它们时需要注意输入数据类型和特定实现细节。
相关问题
GNNExplainer最新版本的torch_geometric怎么调用explain_node()
GNNExplainer是一个用于解释图神经网络模型预测结果的工具,它基于PyTorch Geometric库。在最新版本中,如果你想要使用`torch_geometric`调用`explain_node()`函数,首先你需要安装`gnnexplainer`库,然后按照以下步骤操作:
1. 导入必要的模块:
```python
import torch_geometric.nn as pyg_nn
from gnnexplainer import GNNExplainer
```
2. 准备好你的图数据(包括`data`, `edge_index`和`adj_matrix`等),以及已经训练好的GNN模型(比如GCN、GAT等)。
3. 初始化GNNExplainer对象,传入模型和一些解释器参数,如`eps`(邻域大小)、`alpha`(注意力权重)等:
```python
model = YourTrainedGNNModel()
explainer = GNNExplainer(model, device='cuda', num_features=data.num_features)
```
这里假设你的模型已经在GPU上运行(如果在CPU上则替换为'device=torch.device("cpu")')。
4. 要解释某个节点的预测,提供该节点的ID,并调用`explain_node()`方法:
```python
node_id_to_explain = 0 # 替换为你感兴趣的节点ID
attention_scores, explanation = explainer.explain_node(data.node_ids[node_id_to_explain], edge_index, num_samples=100)
```
`attention_scores`将返回每个邻居节点对目标节点影响的重要性,`explanation`则是生成的局部特征重要性。
dgllife.model.gnn与torch_geometric.nn.GraphConv如何互相转换使用
dgllife和torch_geometric都是流行的图神经网络框架,其中dgllife提供了一个名为gnn的模块,而torch_geometric则提供了一个名为GraphConv的模块。这两个模块的主要作用都是对图数据进行卷积操作。
如果你想在dgllife中使用torch_geometric的GraphConv,可以通过将GraphConv转换为一个DGL GraphConv来实现。具体地说,你需要将GraphConv的权重矩阵转换为DGL GraphConv中的权重张量,然后再将其传递给DGL GraphConv。示例代码如下:
```python
import torch
from dgllife.model import GCN
# 转换torch_geometric的GraphConv为DGL GraphConv
class GraphConv(torch.nn.Module):
def __init__(self, in_channels, out_channels):
super(GraphConv, self).__init__()
self.conv = torch_geometric.nn.GraphConv(in_channels, out_channels)
def forward(self, g, feat):
# 将权重矩阵转换为权重张量
weight = torch.transpose(self.conv.weight, 0, 1)
weight = torch.unsqueeze(weight, dim=0)
weight = weight.repeat(g.batch_size, 1, 1)
# 传递给DGL GraphConv
return torch.relu(dgl.nn.GraphConv(out_channels, out_channels)(g, feat, weight))
# 创建一个包含GraphConv的GCN模型
class GCN(torch.nn.Module):
def __init__(self, in_feats, hidden_feats, out_feats):
super(GCN, self).__init__()
self.gcn_layers = torch.nn.ModuleList()
self.gcn_layers.append(GraphConv(in_feats, hidden_feats))
self.gcn_layers.append(GraphConv(hidden_feats, out_feats))
def forward(self, g, feat):
for i, layer in enumerate(self.gcn_layers):
if i != 0:
feat = torch.relu(feat)
feat = layer(g, feat)
return feat
```
如果你想在torch_geometric中使用dgllife的gnn,可以通过将gnn转换为一个torch_geometric GraphConv来实现。具体地说,你需要将gnn的权重张量转换为GraphConv中的权重矩阵,然后再将其传递给GraphConv。示例代码如下:
```python
import torch_geometric.nn as pyg_nn
# 转换dgllife的gnn为torch_geometric GraphConv
class GNN(torch.nn.Module):
def __init__(self, in_feats, hidden_feats, out_feats):
super(GNN, self).__init__()
self.gnn = dgllife.model.gnn.AttentiveFPGNN(in_feats=in_feats,
hidden_feats=hidden_feats,
out_feats=out_feats,
num_layers=2)
def forward(self, g, feat):
# 将权重张量转换为权重矩阵
weight = torch.transpose(self.gnn.layers[0].fc.weight, 0, 1)
weight = torch.unsqueeze(weight, dim=0)
# 传递给torch_geometric GraphConv
return pyg_nn.GraphConv(in_channels=self.gnn.layers[0].in_feats,
out_channels=self.gnn.layers[0].out_feats,
bias=True)(g, feat, weight)
# 创建一个包含GraphConv的模型
class PyGCN(torch.nn.Module):
def __init__(self, in_feats, hidden_feats, out_feats):
super(PyGCN, self).__init__()
self.gcn_layers = torch.nn.ModuleList()
self.gcn_layers.append(GNN(in_feats, hidden_feats, out_feats))
self.gcn_layers.append(pyg_nn.GraphConv(hidden_feats, out_feats))
def forward(self, data):
x, edge_index = data.x, data.edge_index
for i, layer in enumerate(self.gcn_layers):
if i != 0:
x = torch.relu(x)
x = layer(edge_index, x)
return x
```
需要注意的是,这两种转换方式可能会对模型的性能产生一定的影响,因此在使用时应该进行适当的调整和比较。
阅读全文
相关推荐
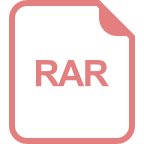
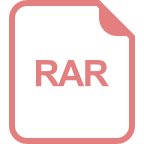
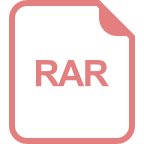
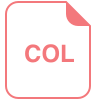
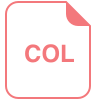











