java实现递归获取json中指定的属性值
时间: 2023-02-22 08:06:59 浏览: 67
在 Java 中,可以使用递归的方法来获取 JSON 中指定的属性值。
首先,需要使用 JSON 库(如 Gson、Jackson)将 JSON 字符串解析成 Java 对象。然后,使用递归算法遍历对象中的所有属性,当找到指定的属性时返回该属性的值。
代码实现如下:
```
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class JsonParserExample {
public static Object getValue(JsonObject jsonObject, String property) {
for (Map.Entry<String, JsonElement> entry : jsonObject.entrySet()) {
if (entry.getKey().equals(property)) {
return entry.getValue();
}
if (entry.getValue().isJsonObject()) {
return getValue(entry.getValue().getAsJsonObject(), property);
}
}
return null;
}
public static void main(String[] args) {
String json = "{\"foo\": {\"bar\": {\"baz\": 42}}}";
JsonElement jsonElement = new JsonParser().parse(json);
Object value = getValue(jsonElement.getAsJsonObject(), "baz");
System.out.println(value);
}
}
```
这段代码使用了 Gson 库,但同样的思路也可以使用其他 JSON 库实现。
相关推荐
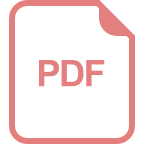
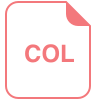














