c# 图形 放大缩小旋转 代码
时间: 2024-11-22 07:46:09 浏览: 4
在 C# 中,图形的放大、缩小和旋转通常涉及到窗口上的图形控件(如PictureBox或Windows Presentation Foundation (WPF) 的 Image 或 UIElement)。这里给出一个基本的例子,使用Windows Forms中的PictureBox和AForge.NET库(用于图像处理):
```csharp
using System.Drawing;
using AForge.Imaging;
public partial class Form1 : Form
{
private Bitmap originalImage; // 原始图片
public Form1()
{
InitializeComponent();
LoadImage(); // 加载图片到PictureBox
}
private void LoadImage()
{
pictureBox1.Image = new Bitmap("path_to_your_image.jpg"); // 替换为实际图片路径
originalImage = (Bitmap)pictureBox1.Image.Clone(); // 保存原始尺寸
}
// 按比例缩放图片
private void zoomPictureBox(float scale)
{
Bitmap scaledImage = new Bitmap(pictureBox1.Width * scale, pictureBox1.Height * scale);
Graphics g = Graphics.FromImage(scaledImage);
g.InterpolationMode = InterpolationMode.HighQualityBicubic;
g.DrawImage(originalImage, 0, 0, scaledImage.Width, scaledImage.Height);
pictureBox1.Image = scaledImage;
}
// 旋转图片
private void rotatePictureBox(float angleInDegrees)
{
RotateFlipType flipType = RotateFlipType.RotateNoneFlipX;
RotateFlip(rotationAngle: angleInDegrees, flipHorizontal: flipType == RotateFlipType.RotateNoneFlipX,
flipVertical: flipType == RotateFlipType.Rotate90FlipXY);
}
// 自定义旋转方法
private void RotateFlip(int rotationAngle, bool flipHorizontal, bool flipVertical)
{
using (Bitmap rotatedImage = new Bitmap(pictureBox1.Width, pictureBox1.Height))
{
Graphics g = Graphics.FromImage(rotatedImage);
g.TranslateTransform(-pictureBox1.Width / 2, -pictureBox1.Height / 2);
g.RotateTransform(rotationAngle);
g.TranslateTransform(pictureBox1.Width / 2, pictureBox1.Height / 2);
if (flipHorizontal || flipVertical)
g.FlipFlip(flipHorizontal, flipVertical);
g.DrawImage(originalImage, new Rectangle(0, 0, rotatedImage.Width, rotatedImage.Height));
pictureBox1.Image = rotatedImage;
}
}
// 示例:触发缩放和旋转事件
private void buttonZoom_Click(object sender, EventArgs e)
{
zoomPictureBox(scaleFactor); // 调整scaleFactor为所需比例
}
private void buttonRotate_Click(object sender, EventArgs e)
{
rotatePictureBox(angleDegree); // 调整angleDegree为所需度数
}
}
```
阅读全文
相关推荐
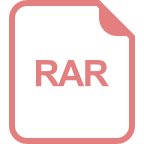
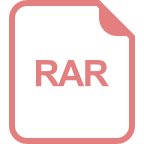
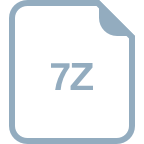
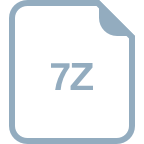
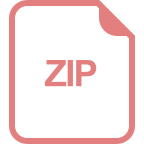
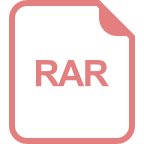
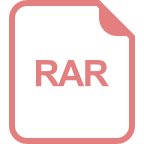
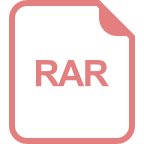
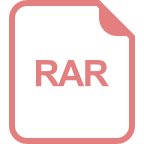
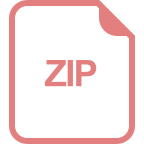
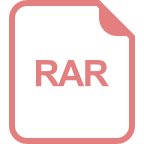
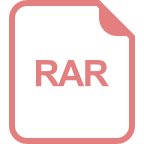
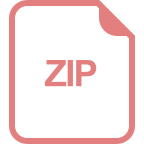
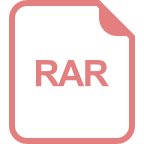
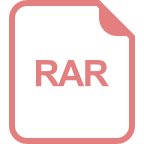
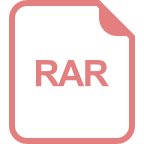
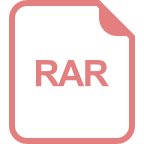
