写一段python代码,实现根据音频名称,将好几段音频拼接
时间: 2024-05-03 20:23:21 浏览: 5
以下为一种实现方法:
```python
import os
import librosa
def concatenate_audio(audio_names, output_name):
# audio_names: a list of audio file names to be concatenated
# output_name: the name of the output concatenated audio file
audio_data = []
sr = None
for audio_name in audio_names:
# load audio data
audio_path = os.path.join('audio_path', audio_name) # replace 'audio_path' with the path of the audio files
data, current_sr = librosa.load(audio_path, sr=None)
# resample to the same sample rate
if sr is None:
sr = current_sr
elif sr != current_sr:
data = librosa.resample(data, current_sr, sr)
audio_data.append(data)
# concatenate audio data
concatenated_data = librosa.util.buf_to_pad(audio_data)
# save concatenated audio
output_path = os.path.join('output_path', output_name) # replace 'output_path' with the path to save the output file
librosa.output.write_wav(output_path, concatenated_data, sr=sr)
```
使用方法:
```python
audio_names = ['audio1.wav', 'audio2.wav', 'audio3.wav']
output_name = 'concatenated_audio.wav'
concatenate_audio(audio_names, output_name)
```
其中,`audio_names`为需要拼接的音频文件名列表,`output_name`为拼接后输出的文件名。需要将代码中的`'audio_path'`和`'output_path'`替换为实际的路径。此外,代码中假设所有音频文件的采样率相同,如有不同需要进行采样率转换。
相关推荐
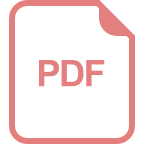
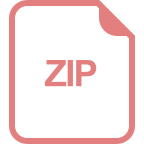
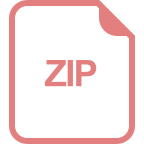














