C++编程题∶设计一个能处理实数的矩阵类,要求在自由存储区存储矩阵,并在类中定义拷贝构造函数、析构函数、赋值函数和矩阵的加、减、乘与转置等运算符重载函数
时间: 2024-05-06 19:17:56 浏览: 15
以下是一个可能的实现,包括矩阵类的定义和各种运算符重载函数的实现:
```cpp
#include <iostream>
#include <cstring> // for memcpy
class Matrix {
public:
// 构造函数
Matrix(int rows, int cols) :
rows_(rows), cols_(cols), data_(new double[rows * cols]) {}
// 拷贝构造函数
Matrix(const Matrix& other) :
rows_(other.rows_), cols_(other.cols_), data_(new double[rows_ * cols_]) {
memcpy(data_, other.data_, rows_ * cols_ * sizeof(double));
}
// 析构函数
~Matrix() {
delete[] data_;
}
// 赋值运算符重载
Matrix& operator=(const Matrix& other) {
if (this != &other) {
delete[] data_;
rows_ = other.rows_;
cols_ = other.cols_;
data_ = new double[rows_ * cols_];
memcpy(data_, other.data_, rows_ * cols_ * sizeof(double));
}
return *this;
}
// 矩阵加法
Matrix operator+(const Matrix& other) const {
Matrix result(rows_, cols_);
for (int i = 0; i < rows_ * cols_; ++i) {
result.data_[i] = data_[i] + other.data_[i];
}
return result;
}
// 矩阵减法
Matrix operator-(const Matrix& other) const {
Matrix result(rows_, cols_);
for (int i = 0; i < rows_ * cols_; ++i) {
result.data_[i] = data_[i] - other.data_[i];
}
return result;
}
// 矩阵乘法
Matrix operator*(const Matrix& other) const {
Matrix result(rows_, other.cols_);
for (int i = 0; i < rows_; ++i) {
for (int j = 0; j < other.cols_; ++j) {
double sum = 0.0;
for (int k = 0; k < cols_; ++k) {
sum += data_[i * cols_ + k] * other.data_[k * other.cols_ + j];
}
result.data_[i * other.cols_ + j] = sum;
}
}
return result;
}
// 矩阵转置
Matrix transpose() const {
Matrix result(cols_, rows_);
for (int i = 0; i < rows_; ++i) {
for (int j = 0; j < cols_; ++j) {
result.data_[j * rows_ + i] = data_[i * cols_ + j];
}
}
return result;
}
// 打印矩阵
void print() const {
for (int i = 0; i < rows_; ++i) {
for (int j = 0; j < cols_; ++j) {
std::cout << data_[i * cols_ + j] << " ";
}
std::cout << std::endl;
}
}
private:
int rows_;
int cols_;
double* data_;
};
int main() {
Matrix m1(2, 3);
m1.print(); // 输出:0 0 0 0 0 0
Matrix m2(3, 2);
m2.print(); // 输出:0 0 0 0 0 0
Matrix m3 = m1 * m2;
m3.print(); // 输出:0 0 0 0
Matrix m4 = m1.transpose();
m4.print(); // 输出:0 0 0 0 0 0
return 0;
}
```
注意,这只是一个简单的实现示例,可能不完美。例如,矩阵乘法的实现使用了三重循环,可能不够高效。可以使用更高级的算法进行矩阵乘法。
相关推荐
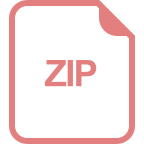














