if (requestCapitalFundsMgr.getFundMoney().equals(capitalFundsMgr.getFundMoney()) && requestCapitalFundsMgr.getFundType().equals(capitalFundsMgr.getFundType()) ) { return getJdbcSharp().update(capitalFundsMgr); } CapitalAccountMgr accountMgr = accountMgrService .findOne(capitalFundsMgr.getAccountId(), capitalFundsMgr.getProjectId()); BigDecimal balance = accountMgr.getBalance(); // 当收支类型与库中数据类型一致时,追加或减少金额 if (requestCapitalFundsMgr.getFundType().equals(capitalFundsMgr.getFundType())) { accountMgr.setBalance(balance.add(requestCapitalFundsMgr.getFundMoney().subtract(capitalFundsMgr.getFundMoney()))); } else { // 当收支类型与库中数据类型不一致时 if (requestCapitalFundsMgr.getFundType() == 0) { // 当支出修改为收入时,计算新余额 余额 = 原始余额 + 新收入 + 原库中支出 BigDecimal newBalance = balance .add(requestCapitalFundsMgr.getFundMoney()) .add(capitalFundsMgr.getFundMoney()); accountMgr.setBalance(newBalance); } else { // 当收入修改为支出时,计算新余额 余额 = 原始余额 - 原库收入 -新支出 BigDecimal newBalance = balance .subtract(requestCapitalFundsMgr.getFundMoney()) .subtract(capitalFundsMgr.getFundMoney()); accountMgr.setBalance(newBalance); } } accountMgrService.update(accountMgr); 优化该代码
时间: 2024-02-14 10:27:07 浏览: 122
在给出优化建议之前,首先需要了解代码的逻辑和目的。以下是对给定代码的优化建议:
1. 使用`Objects.equals()`方法进行对象的相等性比较,而不是使用`equals()`方法。这样可以避免`NullPointerException`。
2. 对于`BigDecimal`对象的比较,应使用`compareTo()`方法,而不是使用`equals()`方法。这样可以确保比较的精确度。
3. 考虑使用条件语句来减少嵌套的深度,并提高代码可读性。
下面是优化后的代码:
```java
if (Objects.equals(requestCapitalFundsMgr.getFundMoney(), capitalFundsMgr.getFundMoney()) &&
Objects.equals(requestCapitalFundsMgr.getFundType(), capitalFundsMgr.getFundType())) {
return getJdbcSharp().update(capitalFundsMgr);
}
CapitalAccountMgr accountMgr = accountMgrService.findOne(capitalFundsMgr.getAccountId(), capitalFundsMgr.getProjectId());
BigDecimal balance = accountMgr.getBalance();
if (Objects.equals(requestCapitalFundsMgr.getFundType(), capitalFundsMgr.getFundType())) {
BigDecimal difference = requestCapitalFundsMgr.getFundMoney().subtract(capitalFundsMgr.getFundMoney());
accountMgr.setBalance(balance.add(difference));
} else {
if (requestCapitalFundsMgr.getFundType() == 0) {
BigDecimal newBalance = balance
.add(requestCapitalFundsMgr.getFundMoney())
.add(capitalFundsMgr.getFundMoney());
accountMgr.setBalance(newBalance);
} else {
BigDecimal newBalance = balance
.subtract(requestCapitalFundsMgr.getFundMoney())
.subtract(capitalFundsMgr.getFundMoney());
accountMgr.setBalance(newBalance);
}
}
accountMgrService.update(accountMgr);
```
通过使用`Objects.equals()`方法和`compareTo()`方法,以及优化条件语句的结构,使得代码更加简洁、可读性更高。同时避免了潜在的`NullPointerException`和精度问题。请注意,根据具体需求,你可能需要进一步调整代码以适应业务逻辑。
相关推荐
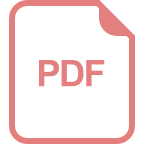
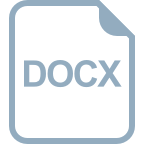














