C++ transpose
时间: 2023-09-02 13:11:11 浏览: 152
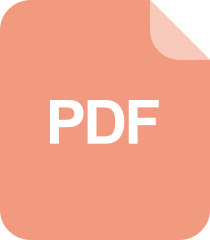
Moving from C to C++
Sure, I can help you with that!
To transpose a matrix in C++, you can use nested loops to iterate over the rows and columns of the matrix. Here's an example code snippet:
```cpp
#include <iostream>
const int ROWS = 3;
const int COLS = 3;
void transposeMatrix(int mat[ROWS][COLS]) {
int transposedMat[COLS][ROWS];
// Transpose the matrix
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
transposedMat[j][i] = mat[i][j];
}
}
// Print the transposed matrix
for (int i = 0; i < COLS; ++i) {
for (int j = 0; j < ROWS; ++j) {
std::cout << transposedMat[i][j] << " ";
}
std::cout << std::endl;
}
}
int main() {
int matrix[ROWS][COLS] = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
std::cout << "Original Matrix:" << std::endl;
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
std::cout << "Transposed Matrix:" << std::endl;
transposeMatrix(matrix);
return 0;
}
```
This code defines a `transposeMatrix` function that takes a 2D array as input and prints its transposed matrix. In the `main` function, we define a sample matrix and print both the original and transposed matrices.
When you run this code, you will get the following output:
```
Original Matrix:
1 2 3
4 5 6
7 8 9
Transposed Matrix:
1 4 7
2 5 8
3 6 9
```
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
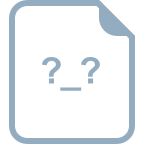


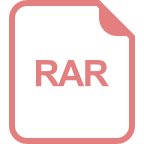
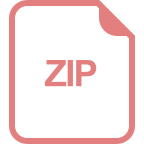
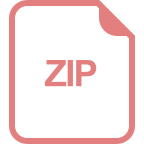
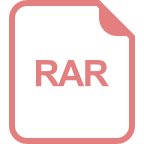
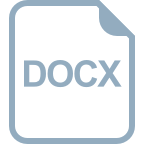







