用c++a) 实现指定 m ,n 参数的函数(要分配数组空间) b) 实现按位置(row,col)赋值函数。 c) 实现求转置函数 Transpose(); Transpose就是行列对换
时间: 2024-05-05 16:23:02 浏览: 114
以下是用 C 实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义矩阵结构体
typedef struct {
int row;
int col;
int **data;
} Matrix;
// 创建矩阵
Matrix *createMatrix(int row, int col) {
Matrix *mat = (Matrix *) malloc(sizeof(Matrix));
mat->row = row;
mat->col = col;
mat->data = (int **) malloc(row * sizeof(int *));
for (int i = 0; i < row; i++) {
mat->data[i] = (int *) malloc(col * sizeof(int));
}
return mat;
}
// 按位置赋值
void setValue(Matrix *mat, int row, int col, int value) {
mat->data[row][col] = value;
}
// 求转置
Matrix *transpose(Matrix *mat) {
Matrix *tMat = createMatrix(mat->col, mat->row);
for (int i = 0; i < mat->row; i++) {
for (int j = 0; j < mat->col; j++) {
tMat->data[j][i] = mat->data[i][j];
}
}
return tMat;
}
// 打印矩阵
void printMatrix(Matrix *mat) {
for (int i = 0; i < mat->row; i++) {
for (int j = 0; j < mat->col; j++) {
printf("%d ", mat->data[i][j]);
}
printf("\n");
}
}
int main() {
// 创建矩阵
Matrix *mat = createMatrix(3, 4);
// 赋值
setValue(mat, 0, 0, 1);
setValue(mat, 0, 1, 2);
setValue(mat, 0, 2, 3);
setValue(mat, 0, 3, 4);
setValue(mat, 1, 0, 5);
setValue(mat, 1, 1, 6);
setValue(mat, 1, 2, 7);
setValue(mat, 1, 3, 8);
setValue(mat, 2, 0, 9);
setValue(mat, 2, 1, 10);
setValue(mat, 2, 2, 11);
setValue(mat, 2, 3, 12);
// 打印矩阵
printMatrix(mat);
// 求转置
Matrix *tMat = transpose(mat);
// 打印转置后的矩阵
printMatrix(tMat);
return 0;
}
```
阅读全文
相关推荐
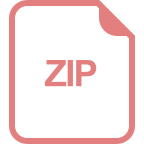








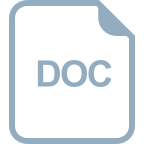







