将矩阵类改为类模板。 //设计矩阵类(m×n); //a) 实现指定 m, n 参数的函数(要分配数组空间) //b) 实现按位置(row, col)赋值函数。 //c) 实现求转置函数 Transpose(); Transpose就是行列对换 //在main函数中验证
时间: 2023-12-20 14:07:42 浏览: 56
好的,我来为您实现一个类模板的矩阵类:
```c++
#include <iostream>
#include <vector>
template <typename T>
class Matrix {
public:
Matrix(int m, int n) : rows(m), cols(n) {
data.resize(rows);
for(int i=0; i<rows; i++) {
data[i].resize(cols);
}
}
void setValue(int row, int col, T value) {
data[row][col] = value;
}
Matrix<T> Transpose() {
Matrix<T> trans(cols, rows);
for(int i=0; i<cols; i++) {
for(int j=0; j<rows; j++) {
trans.setValue(i, j, data[j][i]);
}
}
return trans;
}
void print() {
for(int i=0; i<rows; i++) {
for(int j=0; j<cols; j++) {
std::cout << data[i][j] << " ";
}
std::cout << std::endl;
}
}
private:
int rows, cols;
std::vector<std::vector<T>> data;
};
int main() {
Matrix<int> mat(3, 2);
mat.setValue(0, 0, 1);
mat.setValue(0, 1, 2);
mat.setValue(1, 0, 3);
mat.setValue(1, 1, 4);
mat.setValue(2, 0, 5);
mat.setValue(2, 1, 6);
std::cout << "Original Matrix:" << std::endl;
mat.print();
Matrix<int> trans = mat.Transpose();
std::cout << "Transposed Matrix:" << std::endl;
trans.print();
return 0;
}
```
在上面的代码中,我们使用了类模板来定义一个矩阵类。在类模板中,我们使用了一个类型参数 T,来表示矩阵中元素的类型。然后,我们在构造函数中使用了类型参数 T 来定义了一个二维 vector,来存储矩阵中的元素。
在 setValue 函数中,我们按照指定的行列位置赋值。在 Transpose 函数中,我们按照行列对换的方式,来求出转置矩阵。在 print 函数中,我们输出矩阵中的所有元素。
在 main 函数中,我们首先创建了一个 3x2 的矩阵,并且按照指定的行列位置赋值。然后,我们输出原始的矩阵,并且计算出转置矩阵,并输出转置矩阵。
阅读全文
相关推荐
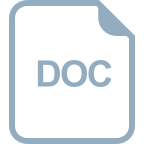
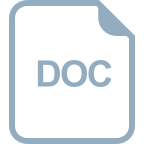
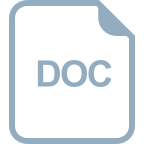



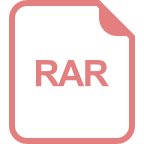
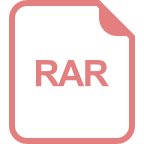
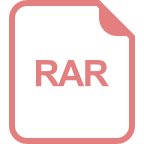
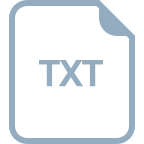
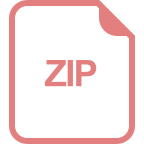
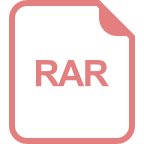
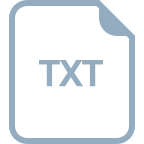
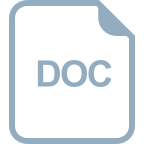
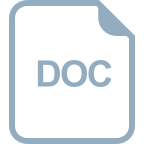
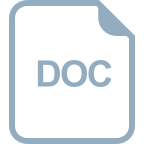
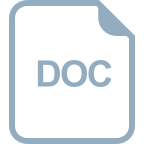
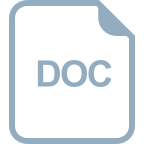
