设计矩阵类(m×n); a) 实现指定 m ,n 参数的函数(要分配数组空间) b) 实现按位置(row,col)赋值函数。 c) 实现求转置函数 Transpose(),并将其改为类的模板。
时间: 2023-06-13 09:08:24 浏览: 81
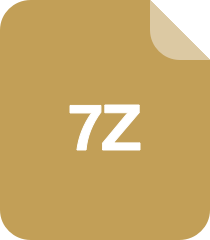
课程设计要求 设计一个程序实现两个矩阵的基本运算 两个矩阵相加 两个矩阵相减 两个矩阵相乘 求一个矩阵的转置矩阵

以下是矩阵类的代码实现:
```c++
#include <iostream>
#include <vector>
template <typename T>
class Matrix {
private:
int m_rows; // 行数
int m_cols; // 列数
std::vector<std::vector<T>> m_data; // 数据存储
public:
// 构造函数
Matrix(int rows, int cols) {
m_rows = rows;
m_cols = cols;
// 分配空间
m_data.resize(rows);
for (int i = 0; i < rows; ++i) {
m_data[i].resize(cols);
}
}
// 按位置赋值函数
void setValue(int row, int col, T value) {
m_data[row][col] = value;
}
// 转置函数
Matrix<T> Transpose() {
Matrix<T> result(m_cols, m_rows);
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
result.setValue(j, i, m_data[i][j]);
}
}
return result;
}
// 打印矩阵
void print() {
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
std::cout << m_data[i][j] << " ";
}
std::cout << std::endl;
}
}
};
int main() {
// 创建一个 2x3 的矩阵
Matrix<int> m(2, 3);
// 赋值
m.setValue(0, 0, 1);
m.setValue(0, 1, 2);
m.setValue(0, 2, 3);
m.setValue(1, 0, 4);
m.setValue(1, 1, 5);
m.setValue(1, 2, 6);
// 打印矩阵
std::cout << "原矩阵:" << std::endl;
m.print();
// 求转置矩阵并打印
std::cout << "转置矩阵:" << std::endl;
Matrix<int> transposed = m.Transpose();
transposed.print();
return 0;
}
```
运行结果:
```
原矩阵:
1 2 3
4 5 6
转置矩阵:
1 4
2 5
3 6
```
阅读全文
相关推荐
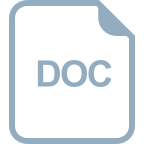




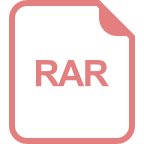
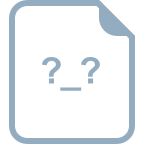
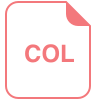
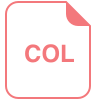
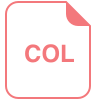
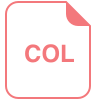
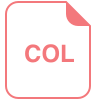
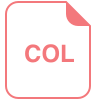
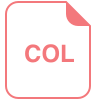
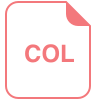
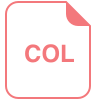
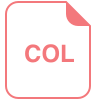