设计矩阵类(m×n) a) 实现指定 m ,n 参数的函数(要分配数组空间) b) 实现按位置(row,col)赋值函数。 c) 实现求转置函数 Transpose(); Transpose就是行列对换
时间: 2024-05-14 09:17:55 浏览: 23
以下是矩阵类的实现代码,包括实现指定 m,n 参数的构造函数,按位置赋值函数 SetValue,以及求转置函数Transpose:
```c++
#include <iostream>
using namespace std;
class Matrix {
private:
int m;
int n;
int** data;
public:
Matrix(int m, int n) {
this->m = m;
this->n = n;
data = new int*[m];
for (int i = 0; i < m; i++) {
data[i] = new int[n];
for (int j = 0; j < n; j++) {
data[i][j] = 0;
}
}
}
void SetValue(int row, int col, int value) {
if (row >= m || col >= n) {
cout << "Invalid index!" << endl;
return;
}
data[row][col] = value;
}
void Transpose() {
int** newData = new int*[n];
for (int i = 0; i < n; i++) {
newData[i] = new int[m];
for (int j = 0; j < m; j++) {
newData[i][j] = data[j][i];
}
}
// 删除原来的数据
for (int i = 0; i < m; i++) {
delete[] data[i];
}
delete[] data;
// 更新成新的数据
data = newData;
int temp = m;
m = n;
n = temp;
}
void Print() {
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
int main() {
Matrix mat(3, 4);
mat.SetValue(0, 0, 1);
mat.SetValue(0, 1, 2);
mat.SetValue(0, 2, 3);
mat.SetValue(0, 3, 4);
mat.SetValue(1, 0, 5);
mat.SetValue(1, 1, 6);
mat.SetValue(1, 2, 7);
mat.SetValue(1, 3, 8);
mat.SetValue(2, 0, 9);
mat.SetValue(2, 1, 10);
mat.SetValue(2, 2, 11);
mat.SetValue(2, 3, 12);
mat.Print();
mat.Transpose();
mat.Print();
return 0;
}
```
在上面的代码中,我们通过构造函数实现了指定 m,n 参数的功能,并在内部分配了数组空间。我们通过 SetValue 函数实现了按位置赋值的功能。Transpose 函数实现了矩阵的转置,即行列对换。在转置函数中,我们创建了一个新的二维数组 newData,将原来的数组 data 的行列互换后存放在 newData 中。接着删除原来的 data 数组,并用 newData 来更新矩阵的数据。最后,我们交换了 m 和 n 的值,以反映行列对换的变化。最后,我们在 main 函数中创建了一个 3x4 的矩阵并进行了一些赋值操作,然后输出原来的矩阵和转置后的矩阵。
相关推荐
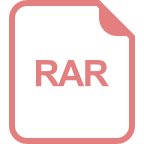
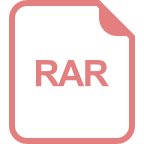
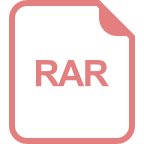














