遗传算法的代码实现python
时间: 2024-05-25 14:09:12 浏览: 22
遗传算法是一种基于生物进化原理的优化算法,主要用于在搜索空间中寻找最优解。它模拟了自然界的生殖、变异和适应过程,通过不断地交叉、变异和选择等操作,逐渐得到更好的解。
以下是一个简单的遗传算法的代码实现,使用Python语言实现:
```python
import random
# 遗传算法实现
def genetic_algorithm(objective_function, chromosome_length, population_size, generation):
# 生成初始种群
population = []
for i in range(population_size):
chromosome = [random.randint(0, 1) for j in range(chromosome_length)]
population.append(chromosome)
# 迭代
for g in range(generation):
# 计算适应度
fitness = [objective_function(chromosome) for chromosome in population]
# 选择
new_population = []
for i in range(population_size):
chromosome1 = roulette_wheel_selection(population, fitness)
chromosome2 = roulette_wheel_selection(population, fitness)
# 交叉
child = crossover(chromosome1, chromosome2)
# 变异
mutate(child)
new_population.append(child)
population = new_population
# 返回最优解
return max(population, key=lambda chromosome: objective_function(chromosome))
# 轮盘赌选择
def roulette_wheel_selection(population, fitness):
total_fitness = sum(fitness)
r = random.uniform(0, total_fitness)
for i in range(len(population)):
r -= fitness[i]
if r <= 0:
return population[i]
# 交叉
def crossover(chromosome1, chromosome2):
point = random.randint(1, len(chromosome1) - 1)
child = chromosome1[:point] + chromosome2[point:]
return child
# 变异
def mutate(chromosome):
point = random.randint(0, len(chromosome) - 1)
chromosome[point] = 1 - chromosome[point]
# 示例目标函数,求最大值
def objective_function(chromosome):
x = int("".join(str(bit) for bit in chromosome), 2)
return x * x
# 测试遗传算法
chromosome_length = 8
population_size = 10
generation = 100
result = genetic_algorithm(objective_function, chromosome_length, population_size, generation)
print(result)
```
上述代码实现了一个简单的遗传算法,其中`objective_function`为目标函数,`chromosome_length`为染色体长度,`population_size`为种群大小,`generation`为迭代次数。
实现过程中使用了轮盘赌选择、交叉和变异等操作,其中轮盘赌选择用于选择优秀的个体,交叉用于产生新的个体,变异用于增加种群的多样性。
在测试中使用了一个简单的目标函数,即求解$x^2$的最大值。
相关推荐
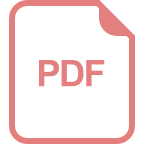
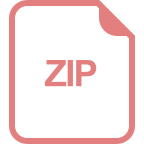














